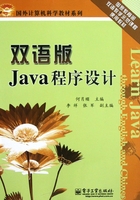
3.7 Composite Data Types
The Java language does not support structs or unions. Instead, classes or interfaces are used to build composite types. In defense of this limitation, classes provide the means to bundle data and methods together, as well as a way to restrict(限制)access to the private data of the class. For example, using a struct in C, we might define a car this way:
struct Car
{
char*model;
char*make;
int year;
Engine*engine;
Body*body;
Tire**tires;
}
The example above implies that we have previously defined Engine, Body, and Tire structs. In Java, the example above assumes that we have previously defined the CarModels and CarMakes interfaces and that we have previously defined the classes Engine, Body, Tire, DurangoEngine, DurangoBody, and GoodyearTire.
3.7.1 Initializing Composite Data Types
Composite data types are initialized by the code defined in the constructor called when a creation technique, such as “new,” is applied to a variable of a class type. Fields of a class are initialized with their default values or with explicit value assignments before the constructor is called.
复合数据类型的初始化由构造方法的代码实现,构造方法是在new创建对象。
In Listing B, when the instance of myClass is created using the new operator, the constructor, public MyClass( ), is called to allow the class to initialize itself. The initialization process occurs as follows:
(1)The statement “int myInt;” is called and assigns the default value, 0, to myInt.
(2)The statement “AnotherClass anotherClass;” is called and assigns the default value, null, to anotherClass.
(3)The statement within the constructor “myint = 2;” is called and assigns the value 2 to myInt.
3.7.2 Predefined Composite Data Types
Java supplies a vast array of predefined composite data types(预定义复合数据类型). One of these is the String class belonging to the java.lang package. The String class provides methods to perform a number of commonly used string operations, such as length( ), substring(int beginIndex), toUpperCase( ), toLowerCase( ), equals( ) and others. Another commonly used composite data type Java provides is the Vector class belonging to the java.util package. The Vector class defines methods to perform commonly used operations on an expandable array of objects. Some of these methods are add(int index, Object element), elementAt(int index), isEmpty( ), and remove(int index). These are a small sample of the predefined composite data types that Java supplies. In future articles, we’ll discuss these and other predefined types in detail.