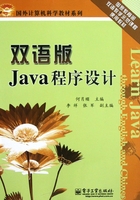
3.6 Complex Data Types
3.6.1 Reference Data Types
Along with primitive data types, the Java Virtual Machine (JVM) defines data types known as reference data types. Reference types “refer” to variables and, even though Java doesn’t have an explicit pointer type, can be thought of as pointers to the actual value or set of values represented by variables. An object can be “pointed to” by more than one reference. The JVM never addresses objects directly; instead, the JVM operates on the objects’ references.
There are three distinct reference types: class, interface, and array. Reference types can contain references to dynamically created instances of classes, instances, and arrays. References may also contain a special value known as the null reference. The null reference has no type at runtime but may be cast to any reference type. The default value for reference types is null.
引用类型被认为是实际值的指针或变量的指针。一个对象可以拥有多个引用。有三种不同的引用类型:类、接口和数组。引用类型能够指向动态创建的类实例、接口和数组。
3.6.2 Class Types
Classes refer to data types that define methods and data. Internally, the JVM typically implements a class type object as a set of pointers to methods and data. Variables defining class types can refer only to instances of the class types or to the null reference, as the following snippet demonstrates:
MyObject anObject = new MyObject(); // 合法 MyObject anotherObject = null; // 合法 MyObject stillAnotherObject = 0; // 不合法
3.6.3 Interface Types
An interface acts as a template defining methods that an object must implement in order to be referred to as an instance of the interface. An interface cannot be instantiated. A class can implement multiple interfaces and be referred to by any of the interfaces that it implements. An interface variable can refer only to instances of classes that implement that interface.
接口扮演着方法模板的角色,对象通过引用接口来实现接口中的方法。
For example, assuming we have defined an interface called Comparable and a class called SortItem, which implements Comparable, we can define the following code:
Comparable c = new SortItem();//对象SortItem实现接口Comparable
If the Comparable interface defines one method, public void compare(Comparable item), then the SortItem class must provide an implementation of the compare method, as follows:
public class SortItem implements Comparable { public void compare(Comparable item) { ...method implementation here } }
接口是定义方法的模板,对象必须执行该方法的目的是引用接口的实例。接口不能实例化。一个类可以实现多个接口,并且可以引用任何被实现的接口。一个接口变量仅引用执行该接口的类的实例。