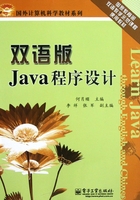
3.8 Casting Variables to a Different Type
There will be occasions when you will have a variable which you wish to store in a variable of another type.
3.8.1 Automatic Casting
When the Java compiler knows that the new data type has enough room to store the new value the casting is automatic and there is no need to specify it explicitly. For example, if you had a value stored in a byte typed variables and wanted to store it in a integer typed variable the compiler will let you because a byte value will always fit in an integer.
int a; // 定义一个变量a byte b=10; // 定义一个字节b并分配10 a = b; // 将b赋值给a
This kind of casting is commonly known as widening since the value has been made wider because it is now stored in a type which is larger than it actually needs, it is useful to view the widened value as padded by zeros.
这种扩展是一种普通的转换,它的值会扩展,因为它存储在一个比它实际还大的类型中,通过填补0来完成这种扩展。
3.8.2 Explicit Casting
When an int value is to be stored in a byte it is possible that the byte will not be able to hold the full value. In this case as explicit casting must be made, the syntax for this is as follows:
variable_of_smaller_type = (smaller_type) value_of larger_type;
该赋值语句将右边较大的类型变量转换为左边类型较小的变量。
At first this appears complicated but it isn’t really, what the code is really saying is that the variable on the left is made equal to the smaller_type version of the value on the right. For the example above, this is:
class CastTest{ public static void main (String args[]){ byte a; //声明一个字节变量a int b=10000; //声明一个整型变量并赋值10000 a = (byte) b; //显式地将b转换成a System.out.println (a); //将a打印出来 } }
In other words the variable a is assigned the byte value of the int variable b. When the full value will not fit in the new type, as in this example, then the value is reduced modulo(模)the byte’s range. In the above example the result stored in a is 16, that is 10000 % 128 = 16, where 128 is the range of a byte(0 to 127). If you want to try this for yourself, the just copy and paste it, save as CastTest.java and compile with the javac command and run with java - as you did for the first program in the last lesson.
将整型变量b的值赋值给字节变量。在这个例子中,变量的值不能完整地装入新的类型中,这个值被缩小为字节型的范围内。