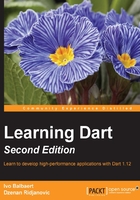
Making a to-do list with Dart
Since this has become the "Hello World" for web programmers, let's make a simple to-do list and start a new todo_v1
web application. To record our tasks, we need an input field corresponding with InputElement
in Dart:
<input id="task" type="text" placeholder="What do you want to do?"/>
The HTML5 placeholder attribute lets you specify a default text that appears in the field.
We specify a list tag (UListElement
), which we will fill up in our code:
<ul id="list"/>
The following is the code from todo_v1.dart
:
import 'dart:html'; InputElement task; UListElement list; main() { task = querySelector('#task'); (1) list = querySelector('#list'); (2) task.onChange.listen( (e) => addItem() ); (3) } void addItem() { var newTask = new LIElement(); (4) newTask.text = task.value; (5) task.value = ''; (6) list.children.add(newTask); (7) }
We bind our HTML elements to the Dart objects task and list in the lines (1)
and (2)
. In line (3)
, we attach an addItem
event handler to the onChange
event of the textfield task: this fires, when the user enters something in the field and leaves it (either by pressing Tab or Enter). UListElement
is, in fact, a collection of LIElements
(these are its children). So, for each new task, we will make LIElement
in (4)
, assign the task's value to it in (5)
, clear the input field in (6)
, and add the new LIElement
to the list in (7)
. In the following screenshot, you can see some tasks to be performed:

A simple to-do list
Of course, this version isn't very useful (unless you want to make a print of your screen); our tasks aren't recorded and we can't indicate the tasks are finished. Don't worry, we will enhance this app in the future versions.