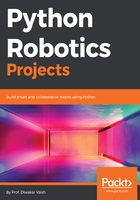
Interfacing the ultrasonic proximity sensor
First, the basics. A proximity sensor is a type of sensor that senses the proximity of an object from it. There is a universe full of sensors that are available to accomplish this task and numerous technologies that allow us to do so. As the name says, the ultrasonic proximity sensor works on the principal of ultrasonic sound waves. The working principle is quite easy to understand. The ultrasonic sensor sends a beam of ultrasonic sound waves; these waves are inaudible to human ears, but nonetheless it is still a sound wave and it also behaves like a sound wave.
Now, as we know, sound bounces off different surfaces and forms an echo. You must have experienced this echo when speaking in an empty room. You can hear your own sound but with a slight delay. This delay is caused by the property of sound. A sound is a wave, hence it has a speed. Sound waves have a set speed of travel. So, to cover a specific distance, they take some time. By calculating this time, we can derive how far the sound waves are going before getting bounced off from a surface.
Similarly, in this sensor, we shoot ultrasonic sound waves in a specific direction and then sense the echo which bounces back. Naturally, there would be a delay in receiving the echo; the delay would be directly proportional to the distance of the object from the sensor and, based on this delay, we could easily compute the distance.
Now, to work with the proximity sensor, we need to understand the physical architecture of the sensor to wire it correctly. There are four pins in the sensor, which are:
- VCC (positive)
- Trigger
- Echo
- GND (ground)
I obviously don't have any need to explain what VCC and ground does. So, let's move on straight to trigger. Whenever the pin is high for 10 microseconds, the ultrasonic sensor will send eight cycles of 40 kHz sound waves to the target. Once the trigger cycle is completed, the ECHO is set to high. Once it receives the echo signal back, the ECHO pin is set back to low. Here is a diagram to show how it actually happens:

That is all we need to know for now. Subsequently, we will learn more as we move along. Now, to go ahead and make it live, connect it as per the diagram:

Once the connection is made, the following is the code that you need to run:
import RPi.GPIO as GPIO
import time
GPIO.setmode(GPIO.BCM)
GPIO.setup(23,GPIO.OUT)
GPIO.setup(24,GPIO.IN)
while True:
pulse_start = 0
pulse_stop = 0
duration = 0
distance = 0
GPIO.output(23,GPIO.LOW)
time.sleep(0.1)
GPIO.output(23,GPIO.HIGH)
time.sleep(0.000010)
GPIO.output(23,GPIO.LOW)
while GPIO.input(24)==0:
pulse_start = time.time()
while GPIO.input(24)==1:
pulse_stop = time.time()
duration = pulse_stop - pulse_start
distance = duration*17150.0
distance = round(distance,2)
print ("distance" + str(distance))
time.sleep(0.2)
}
Now, once you run this program, the output on your screen will be showing you the distance of the object once in every 0.2 seconds. Now, you must be wondering how this is communicating all these readings:
GPIO.setup(23,GPIO.OUT)
We are assigning pin 23 to give pulse to TRIGGER pin of the sensor when required:
GPIO.setup(24,GPIO.IN)
We are assigning pin 24 to receive the logic to confirm the receipt of the echo signal:
pulse_start = 0
pulse_stop = 0
duration = 0
distance = 0
We will be using the preceding as variables, and every time the loop starts we are assigning them a value which is 0; this is to wipe off the previous reading that we would have stored during the course of program:
GPIO.output(23,GPIO.HIGH)
time.sleep(0.000010)
GPIO.output(23,GPIO.LOW)
We keep the trigger pin number 23 high for 0.000010 seconds so that the ultrasonic sensor can send a brief pulse of ultrasonic waves:
while GPIO.input(24)==0:
pulse_start = time.time()
This while statement will keep noting down the time of the pulse_start variable until the time pin number 24 is low. The final reading of the time will be stored in the pulse_start variable, as in noting down the time when the pulse was sent:
while GPIO.input(24)==1:
pulse_stop = time.time()
The while statement in this loop will start noting the time when the input on pin number 24 is high and it will keep noting the time until the pin number 24 remains high. The final reading of the time will be stored in the pulse_stop variable, as in noting down the time when the pulse is received:
duration = pulse_stop - pulse_start
In this statement we are calculating the overall time it took for the pulse to travel from the sensor to the object and bounce back to the receiver on the sensor:
distance = duration*17150.0
This is an arithmetic formula given by the manufacturer to convert the time duration it took for the ultrasonic waves to travel into the actual distance in centimeters. You may ask how did we get to this equation?
Let me give you a brief about it. With elementary physics we would remember this simple equation: Speed = Distance / Time.
Now you may also recall that the speed of sound is 343 meters per second. Now 1 meter has 100 centimeters hence to convert this speed into centimeters per second, we would have to multiply the speed by 100, hence the speed would be 34,300 centimeters per second.
Now we know one element of the equation which is the speed. So lets put the value of speed into the equation. Now the equation would look something like this: 34,300 = Distance / Time.
Now we know one thing that the distance which the sound is travelling is twice the actual distance. How ? Because the sound first goes from the sensor to the object. Then it bounces off that surface and reaches back to the sensor. So essentially it is covering twice the distance. Hence we to adapt this equation we have to make a small change: 34,300 / 2 = Distance / Time
Now what we want out of this equation is distance So lets take all other part to the other side. Now the formula would look something like this: 17,150 * Time = Distance
So here we have it the formula for the distance.
distance = round(distance,2)
As the distance the ultrasonic waves have traveled is twice the actual distance (once for going towards the object and second for bouncing back to the sensor), we pide it by half to get the actual distance:
print 'Distance = ',distance
Finally, we will print the measured distance via the following statement. Anything that is in the quotation marks '...' will be written the way it has been written. However, distance is written without quotation marks, and distance is a variable. Hence, the variable stored in the distance will be written in the final output on the screen:
time.sleep(0.25)
The code will pause on this line for a time of 0.2 seconds. If we did not have this pause, then the values would come out at an incredible speed which would be hard for us to read or understand. If you are tinkering around, I would recommend removing this statement and running the code to see what happens.