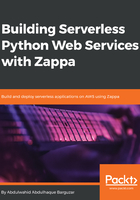
Base model
SQLAlchemy is most famous for its object-relational mapper (ORM), an optional component that provides the data mapper pattern, where classes can be mapped to the database in open-ended, multiple ways, allowing the object model and database schema to develop in a cleanly decoupled way from the beginning. We are using the Flask-SQLAlchemy extension here, which extends the support of SQLAlchemy. Flask-SQLAlchemy enhances the features that may need to be integrated with the Flask application.
We are going to combine the generic SQL operations which are required to interact by using Flask-SQLAlchemy. Hence, we are going to create a base model class and will use this class for other modules' model classes. That's the reason we are putting it under the config directory. Here is the models.py file.
File—config/models.py:
from app import db
class BaseModel:
"""
Base Model with common operations.
"""
def delete(self):
db.session.delete(self)
db.session.commit()
def save(self):
db.session.add(self)
db.session.commit()
return self
You can see here that we group the database operations that are required by all models. The db instance was created in the app/__init__.py file using the Flask-SQLAlchemy extension.
Here, we have implemented the save and delete methods. db.Model defines a generic pattern to create a model class that represents the database table. In order to save and delete, we need to follow some predefined operations such as db.session.add(), db.session.delete(), and db.session.commit().
So, we grouped the generic operations under the save and delete methods. These methods will be called from a model class that will inherit them. We will look at this later when we create a model class by extending BaseModel.