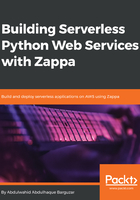
Configuration
While implementing any project, we may be required to have some configurations that are specific to different environments, such as toggling the debug mode in the development environment and monitoring in the production environment.
Flask has a flexible way of overcoming the configuration handling mechanism. Flask provides a config object on its instance. This config object is built by extending the Python dictionary object but with some additional features such as loading the configuration from a file, object, and default built-in configurations. You can look at a detailed description of the config mechanism at http://flask.pocoo.org/docs/0.12/config/.
In order to maintain the configuration based on environments, we are going to create a file called config/config.py with the following code:
import os
from shutil import copyfile
BASE_DIR = os.path.dirname(os.path.dirname(__file__))
def get_sqlite_uri(db_name):
src = os.path.join(BASE_DIR, db_name)
dst = "/tmp/%s" % db_name
copyfile(src, dst)
return 'sqlite:///%s' % dst
class Config(object):
SECRET_KEY = os.environ.get('SECRET_KEY') or os.urandom(24)
SQLALCHEMY_COMMIT_ON_TEARDOWN = True
SQLALCHEMY_RECORD_QUERIES = True
SQLALCHEMY_TRACK_MODIFICATIONS = False
@staticmethod
def init_app(app):
pass
class DevelopmentConfig(Config):
DEBUG = True
SQLALCHEMY_DATABASE_URI = get_sqlite_uri('todo-dev.db')
class ProductionConfig(Config):
SQLALCHEMY_DATABASE_URI = get_sqlite_uri('todo-prod.db')
config = {
'dev': DevelopmentConfig,
'production': ProductionConfig,
}
Here, we created a Config object as a base class that has some generic configuration and Flask-SqlAlchemy configurations. Then, we extended the base Config class with environment-specific classes. Finally, we created a mapping object, which we will use from the aforementioned keys.