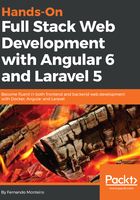
Benefits of TypeScript
Here's a small list of the benefits of using TypeScript:
- TypeScript is robust, secure, and easy to debug.
- TypeScript code is compiled before being transformed into JavaScript, so we can catch all sorts of errors before running the code.
- IDEs that support TypeScript have the ability to improve code completion and checking static typing.
- TypeScript supports OOP (Object Oriented Programming), including modules, namespaces, classes, and more.
The main point in TypeScript's favor is that it has been adopted by the Angular team; and, since Angular is one of the most important frontend frameworks for the development of modern web applications with JavaScript, this has motivated many developers, who are migrating from Version 1.x of the AngularJS to Version 2/4/5/6, to learn it.
A simple reason for this is that the majority of Angular tutorials and examples are written in TypeScript.
- Open sample-01.ts and add the following code, right after the console.log() function:
class MyClass {
public static sum(x:number, y: number) {
console.log('Number is: ', x + y);
return x + y;
}
}
MyClass.sum(3, 5);
- Go back to your Terminal and type the following code:
tsc sample-01.ts
- Now, when you open the sample-01.js file, you will see the results shown in the following screenshot:

Note that the sum class parameters, (x:number, y:number), are given the type number. This is one of the advantages of TypeScript; however, as we are acting according to typing and using numbers within the function call MyClass.sum(3, 5), we cannot see its power.
Let's make a small change, and see the difference.
- Change the MyClass.sum() function call to MyClass.sum('a', 5).
- Go back to your Terminal and type the following command:
tsc sample-01.ts
Note that we receive a TypeScript error:
error TS2345: Argument of type '"a"' is not assignable to parameter of type 'number'.
If you are using VS Code, you will see the message in the following screenshot, before you execute the command to compile the file:

As previously mentioned, VS Code is a powerful editor for the TypeScript language; in addition to having an integrated Terminal, we are able to clearly see the compilation errors.
Instead of typing the same command every time, we can make some modifications to the TS file. We can use the --watch flag, and the compiler will run every change that we make to the file automatically.
- In your Terminal, type the following command:
tsc sample-01.ts --watch
- Now, let's fix it; go back to VS Code and replace the MyClass.sum() function with the following code:
MyClass.sum(5, 5);
To stop the TS compiler, just press Ctrl + C.