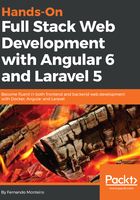
Creating a TypeScript project
Some text editors, such as VS Code, give us the ability to deal with TS files as independent units, called File Scope. Although this is very useful for isolated files (as in the following examples), it is recommended that you always create a TypeScript project. You can then modularize your code and use dependency injection between files in the future.
A TypeScript project is created with a file called tsconfig.json, placed at the root of a directory. You will need to indicate to the compiler which files are part of the project, the compile options, and many other settings.
A basic tsconfig.json file contains the following code:
{ "compilerOptions":
{ "target": "es5",
"module": "commonjs"
}
}
Although the preceding code is very simple and intuitive, we are only indicating which compiler we will use in our project, and also what kind of module. If the code snippet indicates that we are using ECMAScript 5, all TypeScript code will be converted to JavaScript, using ES5 syntax.
Now, let's look at how we can create this file automatically, with the help of the tsc compiler:
- Create a folder, called chapter-02.
- Open your Terminal inside the chapter-02 folder.
- Type the following command:
tsc --init
We will see the following content, generated by the tsc compiler:
{
"compilerOptions": {
/* Basic Options */
/* Specify ECMAScript target version: 'ES3' (default), 'ES5', 'ES2015', 'ES2016', 'ES2017','ES2018' or 'ESNEXT'. */
"target": "es5",
/* Specify module code generation: 'none', 'commonjs', 'amd', 'system', 'umd', 'es2015', or 'ESNext'. */
"module": "commonjs",
...
/* Strict Type-Checking Options */
/* Enable all strict type-checking options. */
"strict": true,
...
/* Enables emit interoperability between CommonJS and ES Modules via creation of namespace objects for all imports. Implies 'allowSyntheticDefaultImports'. */
"esModuleInterop": true
/* Source Map Options */
...
/* Experimental Options */
...
}
}
Note that we have omitted some sections. You should see all of the available options; however, most of them are commented. Do not worry about that at this time; later on, we will look at some of the options in more detail.
Now, let's create a TypeScript file, and check that everything goes smoothly.
- Open VS Code in the chapter-02 folder and create a new file, called sample-01.ts.
- Add the following code to sample-01.ts:
console.log('First Sample With TypeScript');
- Go back to your Terminal and type the following command:
tsc sample-01.ts
Note that another file appears with the same name, but with a .js extension.
If you compare both files, they are exactly the same, because our example is pretty simple, and we are using a simple console.log() function.
As TypeScript is a super set of JavaScript, all of the JS features are available here, too.