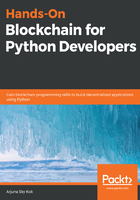
Interacting with smart contracts
Just as we did before, you can use the Truffle console to interact with your smart contract, as follows:
$ truffle console
Your smart contract is always given the name Contract. We can access the smart contract using the following statement:
truffle(development)> Contract.at("0x3E9417399786347B6Ab38f59d3f00829d6bba7b8")
You will get a long output in which you can see abi, bytecode, and so on, as shown in the following screenshot:

Let’s look at the value of the name variable of the smart contract using the following statement:
truffle(development)> Contract.at("0x3E9417399786347B6Ab38f59d3f00829d6bba7b8").then(function(instance) { return instance.name.call(); });
'0x5361746f736869204e616b616d6f746f'
You may notice that the cryptic output does not look like Satoshi Nakamoto. However, it actually is Satoshi Nakamoto, but written in hexadecimal. Let’s throw away 0x from the cryptic output; this is just an indicator that this string is in hexadecimal form. You now have the 5361746f736869204e616b616d6f746f string. Take the first two numbers, which are 53, and convert them into a decimal number. In Python, you can do this as follows:
>>> int(0x53)
83
So, the decimal number is 83. Do you remember the ASCII table? This is a data table that holds the relations between decimal numbers and characters. So, the decimal number 65 represents the character A (capital A) and the decimal number 66 represents the character B (capital B).
So what is the character of the decimal number 83? You can use Python to find out as follows:
>>> chr(83)
'S'
If you do this for all other hexadecimal characters on which each hexadecimal character takes two number characters, it would spell out Satoshi Nakamoto.
Let’s execute another method in this smart contract using the following code:
truffle(development)> Contract.at("0x3E9417399786347B6Ab38f59d3f00829d6bba7b8").then(function(instance) { return instance.say_hello.call(); })
'0x48656c6c6f2c205361746f736869204e616b616d6f746f'
That cryptic output is just Hello, Satoshi Nakamoto.
Let’s change the name as follows:
truffle(development)> Contract.at("0x3E9417399786347B6Ab38f59d3f00829d6bba7b8").then(function(instance) { return instance.change_name(web3.utils.fromAscii("Vitalik Buterin"), { from: "0x6d3eBC3000d112B70aaCA8F770B06f961C852014" }); });
You will get the following as the output:

The value in the from field is taken from one of the accounts in Ganache. You can just look at the Ganache window and choose any account you like.
We cannot send a string directly to the change_name method; we have to convert it to a hexadecimal string with the web3.utils.fromAscii method first.
Now has the name been changed? Let’s find out. Run the following command:
truffle(development)> Contract.at("0x3E9417399786347B6Ab38f59d3f00829d6bba7b8").then(function(instance) { return instance.name.call(); });
'0x566974616c696b204275746572696e'
Yup, the name has been changed. If you transform that hexadecimal string to an ASCII string, you will get Vitalik Buterin.