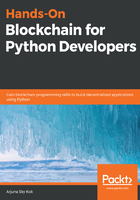
上QQ阅读APP看书,第一时间看更新
Deploying a smart contract to Ganache
So how do you deploy this smart contract to the Ethereum blockchain? There are few ways to do this, but let’s employ a familiar way using Truffle:
- Create a directory and initialize it with truffle init as follows:
$ mkdir hello_project
$ cd hello_project
$ truffle init
- Just as you did in the previous chapter, set truffle-config.js as the following:
module.exports = {
networks: {
"development": {
network_id: 5777,
host: "localhost",
port: 7545
},
}
};
- Create a build directory, as follows:
$ mkdir -p build/contracts
$ cd build/contracts
- Then create a Hello.json file there, as follows:
{
"abi":
"bytecode":
}
- Then fill the abi field with abi or json output from the compilation process, and fill the bytecode field with the bytecode output from the compilation process. You need to quote the bytecode value with double quote marks . Don't forget to put comma between the abi field and the bytecode field. This will give you something similar to the following:
{
"abi": [{"name": "__init__", "outputs": [], "inputs": [], "constant": false, "payable": false, "type": "constructor"}, {"name": "change_name", "outputs": [], "inputs": [{"type": "bytes", "name": "new_name"}], "constant": false, "payable": false, "type": "function", "gas": 70954}, {"name": "say_hello", "outputs": [{"type": "bytes", "name": "out"}], "inputs": [], "constant": false, "payable": false, "type": "function", "gas": 8020}, {"name": "name", "outputs": [{"type": "bytes", "name": "out"}], "inputs": [], "constant": true, "payable": false, "type": "function", "gas": 5112}],
"bytecode": "0x600035601c52740100000000000000000000000000000000000000006020526f7fffffffffffffffffffffffffffffff6040527fffffffffffffffffffffffffffffffff8000000000000000000000000000000060605274012a05f1fffffffffffffffff...
...
1600101808352811415610319575b50506020610160526040610180510160206001820306601f8201039050610160f3005b60006000fd5b61012861049703610128600039610128610497036000f3"
}
- You can then create a migration file to deploy this smart contract by creating a new file in migrations/2_deploy_hello.js, as follows:
var Hello = artifacts.require("Hello");
module.exports = function(deployer) {
deployer.deploy(Hello);
};
After everything is set up, fire up Ganache!
- Then, inside the hello_project directory, you could just run the migration process, as follows:
$ truffle migrate
You will see something similar to the following:

Your smart contract written with Vyper has been deployed to Ganache. Your smart contract address is as follows:
0x3E9417399786347B6Ab38f59d3f00829d6bba7b8