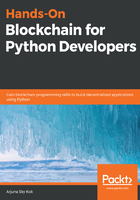
Interacting with smart contracts
To interact with your smart contract that resides in Ethereum blockchain, execute this command inside your Truffle project directory:
$ truffle console
Then, in the truffle console prompt, execute the following command:
truffle(development)> Donation.deployed().then(function(instance) { return instance.useless_variable.call(); });
'Donation string'
If you are confused about then, the Truffle console uses the concept of callback, on which accessing the smart contract object is executed asynchronously. This statement in the Truffle console returns immediately before the callback is being executed. In the callback function, you will accept the smart contract instance as an instance parameter. Then, we can access our useless_variable variable from this instance parameter. Then, to retrieve the value, we have to execute the call method on that variable.
The Truffle framework would use the abi defined in the Donation.json file to understand what interfaces are available in your smart contract. Recall that you define the useless_variable in your smart contract and set it to Donation string in the constructor (or initialization) function. It is free to read a public variable in this way; it does not cost any ether because it is stored in blockchain.
Let me remind you what it means if the variable is stored in blockchain. If you incorporate this smart contract in the Ethereum production blockchain, the useless_variable variable will be stored in every Ethereum node. At the time of writing, there are around 10,000 nodes. This number keeps changing, as can be seen here: https://www.ethernodes.org. A node can be in one computer, and a computer can hold a couple of nodes. However, it is most likely that one computer holds only one node because the requirement to become the host of a node is pretty high. You need a node if you want to interact with the blockchain (there are also other options for this, such as using API to interact with someone else's node). For this reason, it is free to read the useless_variable variable because you just read it from your computer.
If you are confused by this free concept, let's make it clearer by changing the useless_variable variable into something else:
truffle(development)> Donation.deployed().then(function(instance) { return instance.change_useless_variable("sky is blue", {from: "0xb105F01Ce341Ef9282dc2201BDfdA2c26903da77" }); });
You would get the following output:

There is another cryptic hexadecimal number after the word from, which is 0xb105F01Ce341Ef9282dc2201BDfdA2c26903da77. This is the public address of the first account in Ganache. You can confirm it by looking at the Ganache screen. Here, there is a difference in the way you read the useless_variable variable and set it with different content. Changing the content of the variable requires different syntax and, more importantly, an account to use. An account is required because you need to spend some money when changing the variable in blockchain. When you change the value of this useless_variable variable in the smart contract in the blockchain, you are basically broadcasting to all Ethereum nodes in the Ethereum production blockchain, which has around 10,000 nodes available to update the content of useless_variable. We are using Ganache, which is an Ethereum development blockchain, but in a production setting, you need to sign your transaction to change the content of the variable with a private key. A private key's purpose is similar to a password on an account, but a private key cannot be changed, while you can update your password as many times as you like. If you forget your password, you could reset it and click the link in your confirmation email to update it. In blockchain, this is not an option.
If you check Ganache now, your balance stays the same; only the block number increases from 4 to 5:

This happens because the amount of money required is so small. You can look at the output of the command to change the value of useless_variable after you execute the last command. Look at the gas used field; this is what you spent when executing a function in a smart contract. The amount of gas used is 33684, but this is in gwei, not in ether. 1 ether is equal to 1,000,000,000 gwei, so it is around 0.00003 ether. In this case, the gas is calculated automatically but, later, you can set how much gas you want to allocate when executing any function in Ethereum blockchain. If you do not contribute much ether, and the amount of gas allocated is small, there is a big chance your execution will be accorded lower priority. It will take longer for the transaction to be confirmed (meaning the value of the variable has been changed). It could get rejected by miners if the network is experiencing heavy traffic.
This concept of spending money to change the state of the program is new. Reading everything from blockchain is free because all the data is in your computer (if you have Ethereum node), but to change or add something in blockchain requires money. This occurs because you change the data in all Ethereum nodes across the globe, which is expensive! As well as changing the state of the smart contract, computation that runs in memory also requires money.