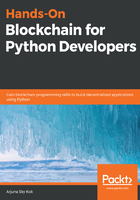
Deploying a smart contract to Ethereum blockchain
These are the steps for deploying a smart contract to Ethereum blockchain with Truffle:
- Write a migration script: To deploy your smart contract, you need to write a migration file. Create a new file named migrations/2_deploy_donation.js. Then, we fill this file with the following script:
var Donation = artifacts.require("./Donation.sol");
module.exports = function(deployer) {
deployer.deploy(Donation);
};
As for the migrations/1_initial_migration.js and contracts/Migrations.sol files, we leave these as they are for now. Truffle needs these files in order to deploy a smart contract.
- Launch Ganache (the blockchain for Ethereum development): Now you need to launch Ganache. Assuming you have been given proper permission, run the following command line to execute the file:
./ganache-1.2.3-x86_64.AppImage
As you can see in the following screenshot, you have numerous accounts, each with a balance of 100 ethers:

One thing you will notice from the Ganache screen is the RPC SERVER, which is located in http://127.0.0.1:7545. This is where your Ethereum blockchain is located in the Truffle project directory.
- Edit the Truffle configuration file: If you open truffle-config.js, the code will look like this after removing the comment lines:
module.exports = {
networks: {
},
mocha: {
},
compilers: {
solc: {
}
}
};
Wipe it out and add the following lines of code to the truffle-config.js file:
module.exports = {
networks: {
"development": {
network_id: 5777,
host: "localhost",
port: 7545
},
}
};
The host and port is taken from the RPC server in the Ganache screen, and network_id is taken from Network ID in the Ganache screen.
- Execute migration scripts: To deploy your smart contract, you can execute it as follows:
$ truffle migrate
The Truffle framework will take your bytecode defined in the Donation.json file and send it to Ethereum blockchain or Ganache. This will provide you with the following output:

In the 2_deploy_donation.js section, take note of the hexadecimal number after the word contract address:, which is 0x3e9417399786347b6ab38f59d3f00829d6bba7b8. This is the smart contract's address, which is similar to the URL of a web application.
If Network is up to date. was output when you tried to deploy your smart contract, you can delete the files inside the build/contracts directory and run this version using the truffle migrate command:
$ truffle migrate --reset
Now, we will take a look at the changes on the Ganache screen:

The most important thing to note is that the first account, 0xb105F01Ce341Ef9282dc2201BDfdA2c26903da77, has lost money. The balance is no longer 100 ETH; it is 99.98 ETH. So where did the 0.02 ETH go? Miners need to be incentivized in order to write your smart contract into the Ethereum blockchain. Note that, the CURRENT BLOCK is no longer 0, but 4. So, 0.02 ETH will be the fee for the miner who successfully puts the block containing your smart contract into the blockchain. But, of course, in this case, there is no miner because we use Ganache, the Ethereum development blockchain. Ganache just simulates the transaction fee by incorporating a smart contract into the blockchain.
If you click the TRANSACTIONS tab, you will see something like this:

You have now created two contracts (Donation and Migrations). Once a smart contract is deployed, unless you apply a method to shut it down, it will be in the blockchain forever. If there is a bug in your smart contract, you cannot patch it. You have to deploy a fixed smart contract in a different address.