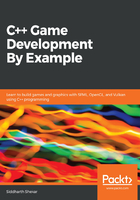
Inheritance
One of the key features of C++ is inheritance, with which we can create classes that are derived from other classes so that the derived or the child class automatically includes some of its parent's member variables and functions.
For example, we looked at the shape class. From this, we can have a separate class called circle and another class called triangle, which has same properties like area as other shapes, such as area.
The syntax for an inherited class is as follows:
class inheritedClassName: accessSpecifier parenClassName{ };
accessSpecifier could be public, private, or protected depending upon the minimum access level you want to provide to the parent member variables and functions.
Let's look at an example of inheritance. Consider the same shape class, which will be the parent class:
class shape { protected: float a, b; public: void setValues(float _length, float _width) { a = _length; b = _width; std::cout << "length is: " << a << " width is: " << b <<
std::endl; } void area() { std::cout << "Area is: " << a * b << std::endl; } };
Since we want the triangle class to access a and b of the parent class, we have to set the access specifier to protected, as shown previously, otherwise, it will be set to private by default. In addition to this, we also change the data type to floats for more precision. After doing this, we create a setValues function instead of the constructor to set the values for a and b. We then create a child class of shape and call it triangle:
class triangle : public shape { public: void area() { std::cout << "Area of a Triangle is: " << 0.5f * a * b <<
std::endl; } };
Due to inheritance from the shape class, we don't have to add a and b member variables, and we don't need to add the setValues member function either, as this is inherited from the shape class. We just add a new function called area, which calculates the area of a triangle.
In the main function, we create an object of the triangle class, set the values, and print the area, shown as follows:
int main() { shape rectangle; rectangle.setValues(8.0f, 12.0f); rectangle.area(); triangle tri; tri.setValues(3.0f, 23.0f); tri.area(); _getch(); return 0; }
Here is the output of this:

To calculate the area of circle, we modify the shape class and add a new overloaded setValues function, as follows:
#include <iostream> #include <conio.h> class shape { protected: float a, b; public: void setValues(float _length, float _width) { a = _length; b = _width; std::cout << "length is: " << a << " height is: " << b <<
std::endl; } void setValues(float _a)
{
a = _a;
} void area() { std::cout << "Area is: " << a * b << std::endl; } };
We will then add a new inherited class, called circle:
class circle : public shape { public: void area() { std::cout << "Area of a Circle is: " << 3.14f * a * a << std::endl; } };
In the main function, we create a new circle object, set the radius, and print the area:
int main() { shape rectangle; rectangle.setValues(8.0f, 12.0f); rectangle.area(); triangle tri; tri.setValues(3.0f, 23.0f); tri.area(); circle c; c.setValues(5.0f); c.area(); _getch(); return 0; }
Here is the output of this:
