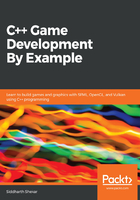
Classes
In C++, structs and classes are identical. You can do absolutely the same thing with both of them. The only difference is the default access specifier: public for structs and private for classes.
The declaration of a class looks like the following code:
class name{ access specifier: name(); ~name(); member1; member2; } ;
A class starts with class keyword, followed by the name of the class.
In a class, we first specify the access specifiers. There are three access specifiers: public, private, and protected:
- public: All members are accessible from anywhere
- private: Members are accessible from within the class itself only
- protected: Members are accessed by other classes that inherit from the class
By default, all members are private.
name(); and ~name(); are called the constructor and destructor of a class. They have the same name as the name of the class itself.
The constructor is a special function that gets called when you create a new object of the class. The destructor is called when the object is destroyed.
We can customize a constructor to set values before using the member variables. This is called constructor overloading.
Notice that, although the constructor and destructor are functions, no return is provided. This is because they are not there for returning values.
Let's look at an example of a class where we create a class called shape. This has two member variables for sides a and b, and a member function, which calculates and prints the area:
class shape { int a, b; public: shape(int _length, int _width) { a = _length; b = _width; std::cout << "length is: " << a << " width is: " << b << std::endl; } void area() { std::cout << "Area is: " << a * b << std::endl; } };
We use the class by creating objects of the class.
Here, we create two objects, called square and rectangle. We set the values by calling the custom constructor, which sets the value of a and b. Then, we call the area function of the object by using the dot operator by pressing the (.) button on the keyboard after typing the name of the object:
int main() { shape square(8, 8); square.area(); shape rectangle(12, 20); rectangle.area(); _getch(); return 0; }
The output is as follows:
