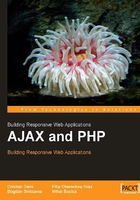
JavaScript Events and the DOM
In the next exercise, we will create an HTML structure from JavaScript code. When preparing to build a web page that has dynamically generated parts, you first need to create its template (which contains the static parts), and use placeholders for the dynamic parts. The placeholders must be uniquely identifiable HTML elements (elements with the ID
attribute set). So far we have used the <div>
element as placeholder, but you will meet more examples over the course of this book.
Take a look at the following HTML document:
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”> <html> <head> <title>AJAX Foundations: More JavaScript and DOM</title> </head> <body> Hello Dude! Here’s a cool list of colors for you: <br/> <ul> <li>Black</li> <li>Orange</li> <li>Pink</li> </ul> </body> </html>
Suppose that you want to have everything in the <ul>
element generated dynamically. The typical way to do this in an AJAX application is to place a named, empty <div>
element in the place where you want something to be generated dynamically:
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”>
<html>
<head>
<title>AJAX Foundations: More JavaScript and DOM</title>
</head>
<body>
Hello Dude! Here’s a cool list of colors for you:
<br/>
<div id=”myDivElement”/>
</body>
</html>
In this example we will use the <div>
element to populate the HTML document from JavaScript code, but keep in mind that you’re free to assign id
s to all kinds of HTML elements. When adding the <ul>
element to the <div>
element, after the JavaScript code executes, you will end up with the following HTML structure:
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”>
<html>
<head>
<title>Colors</title>
</head>
<body>
Hello Dude! Here’s a cool list of colors for you:
<br/>
<div id=”myDivElement”> <ul> <li>Black</li> <li>Orange</li> <li>Pink</li> </ul> </div>
</body>
</html>
Your goals for the next exercise are:
- Access the named
<div>
element programmatically from the JavaScript function. - Having the JavaScript code execute after the HTML template is loaded, so you can access the
<div>
element (no HTML elements are accessible from JavaScript code that executes referenced from the<head>
element). You will do that by calling JavaScript code from the<body>
element’sonload
event. - Group the JavaScript code in a function for easier code handling.
Time for Action—Using JavaScript Events and the DOM
- In the
foundations
folder that you created in the previous exercise, create a new folder calledmorejsdom
. - In the
morejsdom
folder, create a file calledmorejsdom.html
, and add the following code to it:<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”> <html> <head> <title>AJAX Foundations: More JavaScript and DOM</title> <script type=”text/javascript” src=”morejsdom.js”></script> </head> <body onload=”process()”> Hello Dude! Here’s a cool list of colors for you: <br /> <div id=”myDivElement” /> </body> </html>
- Add a new file called
morejsdom.js
, with the following contents:function process() { // Create the HTML code var string; string = “<ul>” + “<li>Black</li>” + “<li>Orange</li>” + “<li>Pink</li>” + “</ul>”; // obtain a reference to the <div> element on the page myDiv = document.getElementById(“myDivElement”); // add content to the <div> element myDiv.innerHTML = string; }
- Load
morejsdom.html
in a web browser. You should see a window like the one in Figure 2.2:Figure 2.2: Your Little HTML Page in Action
What Just Happened?
The code is pretty simple. In the HTML code, the important details are highlighted in the following code snippet:
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”> <html> <head> <title>AJAX Foundations: More JavaScript and DOM</title> <script type=”text/javascript” src=”morejsdom.js”></script> </head> <body onload=”process()”> Hello Dude! Here’s a cool list of colors for you: <br/> <div id=”myDivElement” /> </body> </html>
Everything starts by referencing the JavaScript source file using the <script>
element. The JavaScript file contains a function called process()
, which is used as an event-handler function for the body’s onload
event. The onload
event fires after the HTML file is fully loaded, so when the process()
function executes, it has access to the whole HTML structure. Your process()
function starts by creating the HTML code you want to add to the div
element:
function process() { // Create the HTML code var string; string = “<ul>” + “<li>Black</li>” + “<li>Orange</li>” + “<li>Pink</li>” + “</ul>”;
Next, you obtain a reference to myDivElement
, using the getElementById
function of the document
object. Remember that document
is a default object in JavaScript, referencing the body of your HTML document.
// obtain a reference to the <div> element on the page myDiv = document.getElementById(“myDivElement”);
Note
Note that JavaScript allows you to use either single quotes or double quotes for string variables. The previous line of code can be successfully written like this:
myDiv = document.getElementById(‘myDivElement’);
In the case of JavaScript, both choices are equally good, as long as you are consistent about using only one of them. If you use both notations in the same script you risk ending up with parse errors. In this book, we will use double quotes in JavaScript programs.
Finally, you populate myDivElement
by adding the HTML code you built in the string
variable:
// add content to the <div> element myDiv.innerHTML = string; }
In this example, you have used the innerHTML
property of the DOM to add the composed HTML to your document.