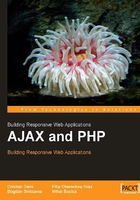
JavaScript and the Document Object Model
As mentioned in Chapter 1, JavaScript is the heart of AJAX. JavaScript has a similar syntax to the good old C language. JavaScript is a parsed language (not compiled), and it has some Object‑Oriented Programming (OOP) capabilities. JavaScript wasn’t meant for building large powerful applications, but for writing simple scripts to implement (or complement) a web application’s client-side functionality (however, new trends are tending to transform JavaScript into an enterprise-class language—it remains to be seen how far this will go).
JavaScript is fully supported by the vast majority of web browsers. Although it is possible to execute JavaScript scripts by themselves, they are usually loaded on the client browsers together with HTML code that needs their functionality. The fact that the entire JavaScript code must arrive unaltered at the client is a strength and weakness at the same time, and you need to consider these aspects before deciding upon a framework for your web solution. You can find very good introductions to JavaScript at the following web links:
- http://www.echoecho.com/javascript.htm
- http://www.devlearn.com/javascript/jsvars.html
- http://www.w3schools.com/js/default.asp
Part of JavaScript’s power on the client resides in its ability to manipulate the parent HTML document, and it does that through the DOM interface. The DOM is available with a multitude of languages and technologies, including JavaScript, Java, PHP, C#, C++, and so on. In this chapter, you will see how to use the DOM with both JavaScript and PHP. The DOM has the ability to manipulate (create, modify, parse, search, etc.) XML-like documents, HTML included.
On the client side, you will use the DOM and JavaScript to:
- Manipulate the HTML page while you are working on it
- Read and parse XML documents received from the server
- Create new XML documents
On the server side, you can use the DOM and PHP to:
- Compose XML documents, usually for sending them to the client
- Read XML documents received from various sources
Two good introductions to DOM can be found at http://www.quirksmode.org/dom/intro.html and http://www.javascriptkit.com/javatutors/dom.shtml. Play a nice DOM game here: http://www.topxml.com/learning/games/b/default.asp. A comprehensive reference of the JavaScript DOM can be found at http://krook.org/jsdom/. The Mozilla reference for the JavaScript DOM is available at http://www.mozilla.org/docs/dom/reference/javascript.html.
In the first example of this chapter, you will use the DOM from JavaScript to manipulate the HTML document. When adding JavaScript code to an HTML file, one option is to write the JavaScript code in a <script>
element within the <body>
element. Take the following HTML file for example, which executes some simple JavaScript code when loaded. Notice the document
object, which is a default object in JavaScript that interacts with the DOM of the HTML page. Here we use its write
method to add content to the page:
<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”> <html> <head> <title>AJAX Foundations: JavaScript and DOM</title> <script type=”text/javascript”> // declaring new variables var date = new Date(); var hour = date.getHours(); // demonstrating the if statement if (hour >= 22 || hour <= 5) document.write(“You should go to sleep.”); else document.write(“Hello, world!”); </script> </head> <body> </body> </html>
The document.write
commands generate output that is added to the <body>
element of the page when the script executes. The content that you generate becomes part of the HTML code of the page, so you can add HTML tags in there if you want.
We advise you try to write well-formed and valid HTML code when possible. Writing code compliant to HTML format maximizes the chances that your pages will work fine with most existing and future web browsers. A useful article about following web standards can be found at http://www.w3.org/QA/2002/04/Web-Quality. You can find a useful explanation of the DOCTYPE
element at http://www.alistapart.com/stories/doctype/. The debate on standards seems to be an endless one, with one group of people being very passionate about strictly following the standards, while others are just interested in their pages looking good on a certain set of browsers. The examples in this book contain valid HTML code, with the exception of a few cases where we broke the rules a little bit in order to make the code easier to understand. A real fact is that very few online websites follow the standards, for various reasons.
You will usually prefer to write the JavaScript code in a separate .js
file that is referenced from the .html
file. This allows you to keep the HTML code clean and have all the JavaScript code organized in a single place. You can reference a JavaScript file in HTML code by adding a child element called <script>
to the <head>
element, like this:
<html>
<head>
<script type=”text/javascript” src=”file.js”></script>
</head>
</html>
Note
Even if you don’t have any code between <script>
and </script>
tags
, don’t be tempted to use the short form <script type=”text/javascript” src=”file.js” />
This causes problems with Internet Explorer 6, which doesn’t load the JavaScript page.
Let’s do a short exercise.
Time for Action—Playing with JavaScript and the DOM
- Create a folder called
foundations
in yourajax
folder. This folder will be used for all the examples in this chapter and the next chapter. - In the
foundations
folder, create a subfolder calledjsdom
. - In the
jsdom
folder, add a file calledjsdom.html
, with the following code in it:<!DOCTYPE html PUBLIC “-//W3C//DTD XHTML 1.1//EN” “http://www.w3.org/TR/xhtml11/DTD/xhtml11.dtd”> <html> <head> <title>AJAX Foundations: JavaScript and DOM</title> <script type=”text/javascript” src=”jsdom.js”></script> </head> <body> I love you! </body> </html>
- In the same folder create a file called
jsdom.js
, and write this code in the file:// declaring new variables var date = new Date(); var hour = date.getHours(); // demonstrating the if statement if (hour >= 22 || hour <= 5) document.write(“Goodnight, world!”); else document.write(“Hello, world!”);
- Load
http://localhost/ajax/foundations/jsdom/jsdom.html
in your web browser, and assuming it’s not late enough, expect to see the message as shown in Figure 2.1 (if it’s past 10 PM, the message would be a bit different, but equally romantic).Figure 2.1: The Hello World Example with JavaScript and the DOM
What Just Happened?
The code is very simple indeed and hence it doesn’t need too many explanations. Here are the main ideas you need to understand:
- Because there is no server-side script involved (such as PHP code), you can load the file in your web browser directly from the disk, locally, instead of accessing it through an HTTP web server. If you execute the file directly from disk, a web browser would likely open it automatically using a local address such as
file:///C:/Apache2/htdocs/ajax/foundations/jsdom/jsdom.html
. - When loading an HTML page with JavaScript code from a local location (
file://
) rather than through a web server (http://
), Internet Explorer may warn you that you’re about to execute code with high privileges (more on security in Chapter 3). - JavaScript doesn’t require you to declare the variables, so in theory you can avoid the
var
keywords. This isn’t a recommended practice though. - The JavaScript script executes automatically when you load the HTML file. You can, however, group the code in JavaScript functions, which only execute when called explicitly.
- The JavaScript code is executed before parsing the other HTML code, so its output is displayed before the HTML output. Notice that “Hello World!”appears before “I love you!”.
One of the problems of the presented code is that you have no control in the JavaScript code over where the output should be displayed. As it is, the JavaScript output appears first, and the contents of the <body>
element come next. Needless to say, this scenario isn’t relevant even to the simplest of applications.
Except for the most simple of cases, having just JavaScript code that executes unconditionally when the HTML page loads is not enough. You will usually want to have more control over when and how portions of JavaScript code execute, and the most typical scenario is when you use JavaScript functions, and execute these functions when certain events (such as clicking a button) on the HTML page are triggered.