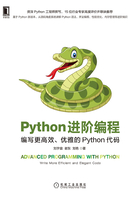
上QQ阅读APP看书,第一时间看更新
4.1.1 手动遍历迭代器
很多开发人员可能习惯使用for循环遍历可迭代对象中的所有元素,这样操作并没有问题,但效率并不是最高的。其实,不用for循环也可以遍历可迭代对象中的所有元素。
我们可使用next()函数遍历可迭代对象并在代码中捕获StopIteration异常。下面展示一个读取文件中所有行的示例,代码如下:
def manual_iter(): with open('/etc/passwd') as f: try: while True: line = next(f) # end 指定为 空,输出行不会有一个空白行 print(line, end='') except StopIteration: pass
上述代码中,StopIteration用来指示迭代的结尾。next()函数可以通过返回一个指定值来标记结尾,如None或空字符,示例如下:
with open('/etc/passwd') as f: while True: line = next(f, None) if not line: break # end 指定为 空,输出行不会有一个空白行 print(line, end='')
很多时候,我们会习惯使用for循环语句来遍历一个可迭代对象。但当需要对迭代做更加精确的控制时,了解底层迭代机制就显得尤为重要了。
下面示例演示了迭代的一些基本细节,代码(hands_iter.py)如下:
num_list = [1, 2, 3] items = iter(num_list) for i in range(len(num_list)): print(f'first items next is:{next(items)}') for i in range(len(num_list) + 1): print(f'second items next is:{next(items)}')
执行py文件,输出结果如下:
first items next is:1 first items next is:2 first items next is:3 second items next is:1 second items next is:2 second items next is:3 Traceback (most recent call last): File "/advanced_programming/chapter4/hands_iter.py", line 27, in <module> print(f'second items next is:{next(items)}') StopIteration
比对输出结果,查看第一个循环输出和第二个循环输出的结果,可以发现第二个循环抛出异常了。