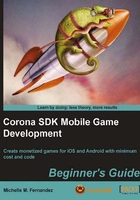
Understanding Corona physics API
Corona has made it convenient to add physics to your games, especially if you've never worked on one before. The engine uses Box2D and takes only a few lines to incorporate it into your application than what it normally takes to have it set up.
Working with the physics engine in Corona is fairly easy. You use display objects and set them as a physical body in your code. Images, sprites, and vector shapes can be turned into a physical object. This is substantial in visualizing how you want your objects to react in an environment you have created. You can see results right away rather than guessing how they might act in a physical world.
Setting up the physics world
Making the physics engine available in your app requires the following line:
local physics = require "physics"
There are three main functions that affect the physics simulation:
This function returns the x and y parameters of the global gravity vector, in units of meter per second square (acceleration units). The default is (0, 9.8) to simulate standard Earth gravity, pointing downwards on the y-axis. For example,
Syntax: physics.setGravity(gx, gy)
physics.setGravity( 0, 9.8 ): Standard Earth gravity
This function returns the x and y parameters of the global gravity vector, in units of meter per second square (acceleration units).
When you have physics.setGravity(gx, gy) and accelerometer API applied, implementing tilt-based dynamic gravity is simple. The following is an example of creating the tilt-based function:
function movePaddle(event) paddle.x = display.contentCenterX - (display.contentCenterX * (event.yGravity*3)) end Runtime:addEventListener( "accelerometer", movePaddle )
The accelerometer is not present in the Corona Simulator; a device build must be created to see the effect.
This function sets the internal pixels-per-meter ratio used in converting between the onscreen Corona coordinates and simulated physics coordinates. This should be done before any physical objects are instantiated.
The default scaling value is 30. For devices of higher resolution like iPad, Android, or iPhone 4, you may wish to increase this value to 60 or more.
Syntax: physics.setScale( value )
physics.setScale( 60 )
There are three rendering modes for the physics engine. This can be altered at any time.
Syntax: physics.setDrawMode( mode )
The physics data is displayed using color-coded vector graphics, which reflect different object types and attributes:
- Orange—dynamic physics bodies (the default body type)
- Dark blue—kinematic physics bodies
- Green—static physics bodies such as the ground or walls
- Gray—a body that is sleeping due to lack of activity
- Light blue—joints
This function sets the accuracy of the engine's position calculations. The default value is 8
, meaning that the engine will iterate through eight position approximations per frame for every object but will increase processor engagement, so it should be handled carefully because it might slow down the application.
Syntax: physics.setPositionIterations( value )
This function sets the accuracy of the engine's velocity calculations. The default value is 3
, meaning that the engine will iterate through three velocity approximations per frame for every object but will increase processor engagement, so it should be handled carefully because it might slow down the application.
Syntax: physics.setVelocityIterations( value )
physics.setVelocityIterations( 6 )