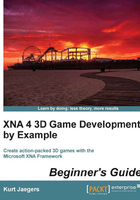
Time for action – the View matrix
- Add the following variable to the
Fields
region of theCamera
class:private Matrix cachedViewMatrix;
- Add the following property to the
Properties
region of theCamera
class:public Matrix View { get { if (needViewResync) cachedViewMatrix = Matrix.CreateLookAt( Position, lookAt, Vector3.Up); return cachedViewMatrix; } }
What just happened?
We could simply recalculate the View
matrix every time the Camera
class was asked for it, but doing so would incur a small performance penalty. Because we do not have a lot of action happening in Cube Chaser, this penalty would not impact our game, but we can avoid it altogether. We are building a caching mechanism into the camera code in the event our game develops to the point that this optimization is helpful. Any time the View
matrix is calculated, we will store it in cachedViewMatrix
and simply return that matrix if the View
matrix is requested without the underlying camera information having been modified.
In order to create the View
matrix, we use the convenient Matrix.CreateLookAt()
method, which accepts the camera position, the look at point we calculated previously, and a vector indicating what direction is considered to be up for the camera. In our case, we are using the pre-defined Vector3.Up
, which translates to (0, 1, 0), or up along the positive Y axis.
That is enough of the camera to get us started. We will return to the Camera
class later when we implement movement. For now, let's get on with actually drawing something to the screen!