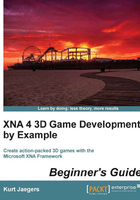
Time for action – beginning the Camera class
- Add a new class to the Cube Chaser project by right-clicking on the project in Solution Explorer and selecting Add | Class....
- Ensure that the Visual C# | Code is selected under Installed Templates and select the Class template.
- Enter
Camera.cs
as the name of the class file. - Add the following
using
directive to the top of theCamera.cs
file:using Microsoft.Xna.Framework;
- Add the following fields and properties to the
Camera
class:#region Fields private Vector3 position = Vector3.Zero; private float rotation; #endregion #region Properties public Matrix Projection { get; private set; } #endregion
- Add a constructor for the
Camer
a
class:#region Constructor public Camera( Vector3 position, float rotation, float aspectRatio, float nearClip, float farClip) { Projection = Matrix.CreatePerspectiveFieldOfView( MathHelper.PiOver4, aspectRatio, nearClip, farClip); MoveTo(position, rotation); } #endregion
What just happened?
We have now put together some of the basic information our Camera
class will track, including the position of the camera and the angle it is facing.
Tip
What is an FPS (First Person Shooter) camera?
When working with cameras in 3D, there are several different kinds of cameras we might want to deal with. An FPS camera, so named because it is the style of camera used in First Person Shooter games, it is a camera that is placed in the location of the character's eyes, resulting in a first-person view. As the player moves, the camera moves and rotates directly with the player. Other types of cameras include chase cameras, which follow along behind the player, and arc-ball cameras, which circle around a fixed point in 3D space. We will implement an arc-ball camera in Chapter 5, Tank Battles – A War-Torn Land.
Additionally, we have defined a matrix, called Projection
, which we will create based on the values passed into the constructor for the Camera
class. It is defined as a property that can be obtained from outside our code, but can only be set within the Camera
class itself.
Tip
What is a matrix?
The short answer is that a matrix is a two-dimensional array of numbers used to transform points in 3D space. The long answer to this question is presented in Chapter 4, Cube Chaser – Finding Your Way, where we will dive into details about what a matrix is and the "magic" behind how they work. For now, we can define a matrix as a construct that we can use to manipulate points by applying different effects to them.
We have not yet defined the MoveTo()
method, which will allow us to specify the camera position and rotation in a single call. We need a few more elements in our class before we can implement MoveTo()
.
Also, note that we have enclosed sections of our code in #region...#endregion
directives. These tell the Visual Studio IDE that these sections of the code can be collapsed as a unit to hide the details while we work on other sections of the code. We will use these regions throughout the book to keep our code organized.