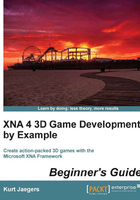
Time for action – customizing the Update() method
Add the following code snippet to the Update()
method before base.Update()
:
Vector2 moveDir = Vector2.Zero; KeyboardState keyState = Keyboard.GetState(); if (keyState.IsKeyDown(Keys.Up)) moveDir += new Vector2(0, -1); if (keyState.IsKeyDown(Keys.Down)) moveDir += new Vector2(0, 1); if (keyState.IsKeyDown(Keys.Left)) moveDir += new Vector2(-1, 0); if (keyState.IsKeyDown(Keys.Right)) moveDir += new Vector2(1, 0); if (moveDir != Vector2.Zero) { moveDir.Normalize(); moveDirection = moveDir; } playerPosition += (moveDirection * playerSpeed * (float)gameTime.ElapsedGameTime.TotalSeconds); playerPosition = new Vector2( MathHelper.Clamp( playerPosition.X, 0, this.Window.ClientBounds.Width - 16), MathHelper.Clamp( playerPosition.Y, 0, this.Window.ClientBounds.Height - 16)); CheckCollisions(); CheckForNewWord();
What just happened?
During each frame, we will begin by assuming that the player is not pressing any movement keys. We create a Vector2
value called moveDir
and set it to the predefined value of Vector2.Zero
, meaning that both the x and y components of the vector will be zero.
In order to read the keyboard's input to determine if the player is pressing a key, we use the Keyboard.GetState()
method to capture a snapshot of the current state of all the keys on the keyboard. We store this in the keyState
variable, which we then use in a series of if
statements to determine if the up, down, left, or right arrow keys are pressed. If any of them are pressed, we modify the value of moveDir
by adding the appropriate vector component to its current value.
After all the four keys have been checked, we will check to see if the value is still Vector2.Zero
. If it is, we will skip updating the moveDirection
variable. If there is a non-zero value in moveDir
, however, we will use the Normalize()
method of the Vector2
class to divide the vector by its length, resulting in a vector pointing in the same direction with a length of one unit. We store this updated direction in the moveDirection
variable, which is maintained between frames.
When we have accounted for all of the possible inputs, we update the player's position by multiplying the moveDirection
by playerSpeed
and the amount of time that has elapsed since Update()
was last called. The result of this multiplication is added to the playerPosition
vector, resulting in the new position for the player.
Before we can assume that the new position is ok, we need to make sure that the player stays on the screen. We do this by using MathHelper.Clamp()
on both the X and Y components of the playerPosition
vector. Clamp()
allows us to specify a desired value and a range. If the value is outside the range, it will be changed to the upper or lower limit of the range, depending on which side of the range it is on. By limiting the range between zero and the size of the screen (minus the size of the player), we can ensure that the player's sprite never leaves the screen.
Finally, we call two functions that we have not yet implemented: CheckCollisions()
and CheckForNewWord()
. We discussed CheckForNewWord()
in the LoadContent()
section, but CheckCollisions()
is new. We will use this method to determine when the player collides with a letter and how to respond to that collision (increase or decrease the player's score, advance the spelling of the current word, and so on).