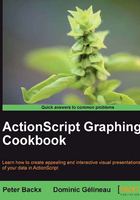
Preparing your data
This first short recipe discusses some general rules that will make your live easier when dealing with graph data.
It also sets you up with a few test files to use throughout the chapter.
Getting ready
Most data in graphs has a tabular form and will usually originate either from a spreadsheet or a database. It's possible to process pretty much any input format with ActionScript 3.0, but some will be easier than others. We will focus on data available in some spreadsheet format. See the There's more... section of this recipe for a discussion on databases.
The spreadsheet examples use Microsoft Excel because it is probably the most wide-spread application, but you can use any other spreadsheet application such as the free OpenOffice, or LibreOffice spreadsheet, or even the spreadsheet functionality in Google Docs.
For CSV (comma separated values) and XML files even a plain text editor, such as Notepad, will suffice.
How to do it...
- Start by creating a text file, named
data.csv
, in whatever text editor you prefer. Enter the following data:0, 20, 50 50, 70, 40 100, 0, 100 150, 150, 150 200, 300, 200 250, 200, 170 300, 170, 160 350, 20, 120 400, 60, 80 450, 250, 150 500, 90, 20 550, 50, 40 600, 110, 90 650, 150, 150 700, 320, 200
Note
Note that, if you are in a country where the decimal separator is a comma and not a dot, you should start from the following file:
0; 20; 50 50; 70; 40 100; 0; 100 150; 150; 150 200; 300; 200 250; 200; 170 300; 170; 160 350; 20; 120 400; 60; 80 450; 250; 150 500; 90; 20 550; 50; 40 600; 110; 90 650; 150; 150 700; 320; 200
- Now open this file in Excel. In the latest version, this should work automatically and you should see the data in three columns. If that doesn't work, use the
import
function to import the CSV file. - Save this file as an Excel workbook (
data.xlsx
). This represents a second source of data. - While Excel can create XML files, it's a complicated process, so we'll just create one in a text editor. Save the following as
data.xml
:<?xml version="1.0" encoding="UTF-8" ?> <data> <row x="0" y1="20" y2="50" /> <row x="50" y1="70" y2="40" /> <row x="100" y1="0" y2="100" /> <row x="150" y1="150" y2="150" /> <row x="200" y1="300" y2="200" /> <row x="250" y1="200" y2="170" /> <row x="300" y1="170" y2="160" /> <row x="350" y1="20" y2="120" /> <row x="400" y1="60" y2="80" /> <row x="450" y1="250" y2="150" /> <row x="500" y1="90" y2="20" /> <row x="550" y1="50" y2="40" /> <row x="600" y1="110" y2="90" /> <row x="650" y1="150" y2="150" /> <row x="700" y1="320" y2="200" /> </data>
- Finally, we will demonstrate how to read files from the Internet. If you have a web server, you can place the
data.csv
file there. Another option is to use Dropbox's public folder: place thedata.csv
file in yourpublic
folder. Right-click on the file and choose Dropbox|Copy public link. This will place a link to the file on the clipboard. - We will also show how to use web services for input. For this recipe, we will use the Yahoo! Weather Service, available at http://developer.yahoo.com/weather/. An example XML file can be found at http://weather.yahooapis.com/forecastrss?w=2502265.
How it works...
As you may have noticed, you will be able to use virtually any input format; although some will be more difficult than others.
Most important is that you keep related variables together. For instance, if an x value of 10 corresponds with an y value of 20, it is not enough to just have the two numbers in the file; you also need to have a way to indicate that they are related.
In your program, you will need to create what is known as a parser, which reads the input file and converts it into a usable ActionScript data structure (as we explained in the first chapter). ActionScript 3.0 comes with parsers for XML and also JSON since Flash Player 11.0. We will see more on JSON when interacting with web services.
The CSV file puts relations on the same line. The file shown in the recipe represents the same data that was used in the previous chapter.
CSV is one of the oldest and easiest data formats. However, on many occasions programs do not follow the RFC 4180 standard and that might create a few interoperability issues. We already saw one such issue: In countries with the comma as decimal separator, you should use the semicolon as data separator for most programs. For instance, a line with decimal data will look like the following format:
10; 20,5; 10,95
While in the US and many other countries it would look like the following format:
10, 20.5, 10.95
You may also need to adapt some of the code in the next recipes to adapt to this situation. By default ActionScript assumes the decimal separator is a point, so you will need to do some conversion on those decimals.
XML files hold the data inside tags and attributes. In contrast to the CSV files, you have much more freedom. The recipe shows one possibility.
Although XML does not force you to use a specific decimal number convention, it's usually best to use the point as separator. Most of the related XML technologies do expect a point.
There's more...
There are many more ways to store data. In the end, much of it is down to personal preference and your specific needs, but some of it will also involve ease of use.
One important data source we have not discussed is databases. The most popular format is the relational database, which stores its data in tables. From a birds-eye view, those tables are somewhat comparable to the spreadsheet worksheets. But there are major differences.
Usually databases are used on servers and are not directly accessed by ActionScript code. This is also the reason why ActionScript has little direct support for databases and why they are not further discussed in this chapter.
The suggested way to deal with databases is to create a REST or SOAP service in a server-side programming language such as PHP, Java, or C# and use that service to read the data from your ActionScript program.