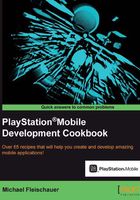
Using the Input2 wrapper class
In the previous recipe, you may have found the bit masking process a little crude. Apparently so did Sony, as they provided a helper method in the GameEngine2D
library named Input2
that makes dealing with input a bit smoother. In this recipe, we are going to demonstrate how that class works.
Getting ready
Open or duplicate the code you created in the previous recipe. Only the contents of the while
loop are going to change in this recipe. The full code for this recipe is available at Ch2_Example2
.
How to do it...
Open AppMain.cs
and change the contents to the following code:
while(!quitApp) { Director.Instance.Update(); string currentStatus = "Your DPad is current pointing at: x="; currentStatus += Input2.GamePad0.Dpad.X; currentStatus += " y="; currentStatus += Input2.GamePad0.Dpad.Y; label.Text = currentStatus; if(Input2.GamePad0.Cross.Press) quitApp = true; if(Input2.GamePad0.Circle.Release) quitApp = true; if(Input2.GamePad0.Square.Down) quitApp = true; if(Input2.GamePad0.Triangle.On) quitApp = true; Director.Instance.Render(); Director.Instance.GL.Context.SwapBuffers(); Director.Instance.PostSwap(); }
Now if you run the app, you will see the following output:

Move the d-pad in different directions and the x and y location will be displayed. You can press any face button to quit.
How it works...
This code simply gets the status of the d-pad and quits if either the Cross
button is pressed, the Circle
button is released, the Square
button is down, or the Triangle
button is On
. The rest of the code is the same boilerplate code you need to work with GameEngine2D
. So what exactly is different here?
Well first off, the d-pad is no longer represented as four independent buttons. Instead it is represented as a normalized 2D vector indicating which direction it is pointing. Pressing the up and left arrow will give you a value of (-1,-1), pressing no buttons will give a value of (0,0), and pressing the down and right arrow will give you a value of (1,1). This allows you to treat the d-pad as a single entity instead of as four separate buttons.
Additionally, all of the various button states we saw in the earlier recipe are still there. Using Input2
they are instead represented at a series of bool values, making the code slightly less compact, but certainly more readable. Keep in mind; this is basically just a helper layer over the top of the standard input functionality. The Input2
helper class provides no new functionality or information, it is simply easier to use.
Finally GamePad.GetInput(0)
has the equivalent shortcut reference Input2.GamePad0
, because as of this point in time you will never have more than one controller.
There's more...
The Input2
helper class is a static global variable. It is available for use once you have referenced GameEngine2D.Base
.
You can also call SetData()
and override the values returned by GetData()
. This can be useful when you want to programmatically set controls, such as during testing, remapping controls dynamically or driving a replay. You simply set a GamePadData
structure to the values you want and pass it into SetData()
.
See also
- See the Handling the controller's d-pad and buttons recipe for the code this recipe is based on, as well as to see the way input is handled without the
Input2
wrapper to help