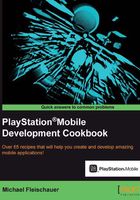
Working with the filesystem
This recipe illustrates the various ways you can access the filesystem.
Getting ready
The complete code for this example is available in Ch1_Example5
. This example adds the following two new using
statements:
using System.Linq;
using System.IO;
How to do it...
Create a new solution and enter the following code replacing the Main()
function:
public static void Main (string[] args){ const string DATA = "This is some data"; byte[] persistentData = PersistentMemory.Read(); byte[] restoredData = persistentData.Take(DATA.Length * 2).ToArray(); string restoredString = System.Text.Encoding.Unicode.GetString(restoredData); byte[] stringAsBytes = System.Text.Encoding.Unicode.GetBytes(DATA); for(int i = 0; i < stringAsBytes.Length; i++) persistentData[i] = stringAsBytes[i]; PersistentMemory.Write (persistentData); using(FileStream fs = File.Open("/Documents/Demo.txt",FileMode.OpenOrCreate)){ if(fs.Length == 0) fs.Write(stringAsBytes,0,stringAsBytes.Length); else{ byte[] fileContents = new byte[fs.Length]; fs.Read(fileContents,0,(int)fs.Length); } } }
How it works...
The first part of this sample demonstrates using the PersistentMemory
class. It is a statically available class, so you cannot allocate one. Instead you access it using PersistentMemory.Read()
. This returns a byte array that you can now modify. We read some data using the LINQ extension Take()
method. We multiplied the size by 2 because the string is stored as UNICODE, which requires 2 bytes per character. We then convert those bytes into a Unicode string. Next, we convert our DATA
string into a byte array, then write it to persistentData
byte by byte in a for
loop. Finally we commit our changes to persistentMemory
by calling PersistentMemory.Write()
, passing in our updated persisitentMemory
byte array.
The next part of the recipe demonstrates traditional file access using the standard .NET
libraries, in this case File
and FileStream
. We open a text file in the /Documents
folder named Demo.txt
, creating it if it doesn't exist. If the file doesn't exist, we write our byte array stringAsBytes
to the file. Otherwise, we read the file contents into a new byte array fileContents
.
The first time you run this example, PersistentMemory.Read()
will contain gibberish, as nothing has been written to it yet. On the second run, it will start with the bytes composing your DATA
string.
There's more...
Persistent storage is limited to 64 KB in space. It is meant for quickly storing information, such as configuration settings. It is physically stored on the filesystem in the /save
directory in a file named pm.dat
.
There are three primary locations for storing files:
/Application
: This is where your application files reside, as well as files added with the "Content" build action. These files are read only./Documents
: This is where you put files that require read and write access./Temp
: This is where you put temporary files, that can be read and written. These files will be erased when your program exits.
The PlayStation Mobile SDK limits directory structures to at most 5 levels deep, including the filename. Therefore, /documents/1/2/3/myfile.txt
is permitted, but /documents/1/2/3/4/myfile.txt
is not.
See also
- See the Loading, displaying, and translating a textured image recipe for details about how to add files to your app's folders using build actions