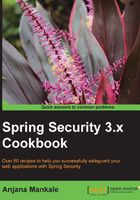
上QQ阅读APP看书,第一时间看更新
Integrating Struts 2 with Spring Security
Let's first set up a Struts 2 application and integrate Spring Security with it.
Getting ready
- Eclipse Indigo or higher version
- JBoss as server
- Struts 2 JARs: 2.1.x
- Spring-core JARs 3.1.4. Release and Spring-Security 3.1.4.Release
- Struts 2 Spring plugin jar
How to do it...
In this section, we will learn how to set up the Struts 2 application with form-based Spring Security:
- In your Eclipse IDE, create a dynamic web project and name it
Spring_Security_Struts2
. - Create a source folder at
src/main/java
. - Create a
struts.xml
file under the source foldersrc/main/java
. - To integrate Struts 2 with the Spring application, add the
application-context.xml
file reference here. - Add the Struts filter mapping in
web.xml
. Spring listener also needs to be added to theweb.xml
file. The listener entry should be above the Struts 2 filter entry. - The
contextLoaderListener
will tell theservletcontainer
about thespringcontextLoader
and it will track events. This also allows the developers to createBeanListeners
, which allow it to track events in the Bean. - In the
web.xml
file, add the following code:<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"> <display-name>Struts2x</display-name> <listener> <listener-class>org.springframework.web.context.ContextLoaderListener</listener-class> </listener> <!—to integrate spring with struts2-> <context-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/applicationContext.xml</param-value> </context-param> <filter> <filter-name>struts2</filter-name> <filter-class>org.apache.struts2.dispatcher.FilterDispatcher</filter-class> </filter> <filter-mapping> <filter-name>struts2</filter-name> <url-pattern>/*</url-pattern> </filter-mapping> </web-app>
- To set up form-based security, we need to create
login.jsp
. The form action isj_spring_security_check
:<%@ taglib prefix="c" url="http://java.sun.com/jsp/jstl/core"%> <html> <head> <title>Login Page</title> <style> .errorblock { color: #ff0000; background-color: #ffEEEE; border: 3px solid #ff0000; padding: 8px; margin: 16px; } </style> </head> <body onload='document.f.j_username.focus();'> <h3>Login with Username and Password (Custom Page)</h3> <% String error=request.getParameter("error"); if(error!=null){ %> <div class="errorblock"> Your login attempt was not successful, try again.<br /> Caused : </div> <%} %> <form name='f' action="<c:url value='/j_spring_security_check'/>" method='POST'> <table> <tr> <td>User:</td> <td><input type='text' name='j_username' value=''> </td> </tr> <tr> <td>Password:</td> <td><input type='password' name='j_password' /> </td> </tr> <tr> <td colspan='2'><input name="submit" type="submit" value="submit" /> </td> </tr> <tr> <td colspan='2'><input name="reset" type="reset" /> </td> </tr> </table> </form> </body> </html>
- Create a folder and name it
secure/hello.jsp
. - Map the
login
action withlogin.jsp
. - Map the
loginfailed
action withlogin.jsp?error=true
. - Map the
welcome
action withsecure/hello.jsp
with the action class-HelloWorld
:struts.xml
:<!DOCTYPE struts PUBLIC "-//Apache Software Foundation//DTD Struts Configuration 2.0//EN" "http://struts.apache.org/dtds/struts-2.0.dtd"> <struts> <package name="default" namespace="/" extends="struts-default"> <action name="helloWorld"> <result>success.jsp</result> </action> <action name="login"> <result>login.jsp</result> </action> <action name="loginfailed"> <result>login.jsp?error=true</result> </action> <action name="welcome" > <result>secure/hello.jsp</result> </action> </package> </struts>
- The
login page
URL is mapped with the Struts 2 action'/login'
. - Security is applied on the Struts 2 action
'/welcome'
. - The user will be prompted to login.
- The user with
role_user
will be authorized to access the pagesApplicationcontext-security.xml
:<beans:beans xmlns="http://www.springframework.org /schema/security" xmlns:beans="http://www.springframework.org /schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://www.springframework.org /schema/beans http://www.springframework.org/schema/beans/spring- beans-3.0.xsd http://www.springframework.org/schema/security http://www.springframework.org/schema/security/spring- security-3.1.xsd"> <global-method-security pre-post-annotations="enabled"> <!-- AspectJ pointcut expression that locates our "post" method and applies security that way <protect-pointcut expression="execution(* bigbank.*Service.post*(..))" access="ROLE_TELLER"/> --> </global-method-security> <http auto-config="true" use-expressions="true" > <intercept-url pattern="/welcome" access="hasRole('ROLE_USER')"/> <form-login login-page="/login" default-target- url="/welcome" authentication-failure- url="/loginfailed?error=true" /> <logout/> </http> <authentication-manager> <authentication-provider> <user-service> <user name="anjana" password="packt123" authorities="ROLE_USER" /> </user-service> </authentication-provider> </authentication-manager> </beans:beans>
How it works...
Just run the application. You will be provided with a link to access the secured page. On clicking on the link you will be prompted to log in. This is actually a form-based login.
Here on submit, the action is sent to the Spring Framework which authenticates the user.
On success, the user will see the authenticated page.
The Struts 2 framework easily gels with the Spring Framework and its modules with very minor modification.



See also
- The Struts 2 application with basic Spring Security recipe
- The Using Struts 2 with digest/hashing-based Spring Security recipe
- The Displaying custom error messages in Struts 2 for authentication failure recipe
- The Authenticating databases with Struts 2 and Spring Security recipe
- The Authenticating with ApacheDS with Spring Security and Struts 2 application recipe
- The Using Spring Security logout with Struts 2 recipe
- The Getting the logged-in user info in Struts 2 with Spring Security recipe