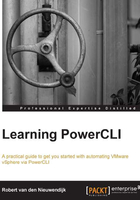
Using objects, properties, and methods
In PowerCLI, even a string is an object. You can list the members of a string object using the Get-Member
cmdlet that you have seen before. Let's go back to our example in Chapter 2, Learning Basic PowerCLI Concepts. First, we create a string "Learning PowerCLI"
and put it in a variable called $String
. Then, we take this $String
variable and execute the Get-Member
cmdlet using the $String
variable as the input:
PowerCLI C:\> $String = "Learning PowerCLI" PowerCLI C:\> Get-Member -Inputobject $String
You can also use the pipeline and do it in a single line, as follows:
PowerCLI C:\> "Learning PowerCLI" | Get-Member
The output will be the following:
TypeName: System.String Name MemberType Definition ---- ---------- ---------- Clone Method System.Object Clone(), Syst... CompareTo Method int CompareTo(System.Object... Contains Method bool Contains(string value) CopyTo Method void CopyTo(int sourceIndex... EndsWith Method bool EndsWith(string value)... Equals Method bool Equals(System.Object o... GetEnumerator Method System.CharEnumerator GetEn... GetHashCode Method int GetHashCode() GetType Method type GetType() GetTypeCode Method System.TypeCode GetTypeCode... IndexOf Method int IndexOf(char value), in... IndexOfAny Method int IndexOfAny(char[] anyOf... Insert Method string Insert(int startInde... IsNormalized Method bool IsNormalized(), bool I... LastIndexOf Method int LastIndexOf(char value)... LastIndexOfAny Method int LastIndexOfAny(char[] a... Normalize Method string Normalize(), string ... PadLeft Method string PadLeft(int totalWid... PadRight Method string PadRight(int totalWi... Remove Method string Remove(int startInde... Replace Method string Replace(char oldChar... Split Method string[] Split(Params char[... StartsWith Method bool StartsWith(string valu... Substring Method string Substring(int startI... ToBoolean Method bool IConvertible.ToBoolean... ToByte Method byte IConvertible.ToByte(Sy... ToChar Method char IConvertible.ToChar(Sy... ToCharArray Method char[] ToCharArray(), char[... ToDateTime Method datetime IConvertible.ToDat... ToDecimal Method decimal IConvertible.ToDeci... ToDouble Method double IConvertible.ToDoubl... ToInt16 Method int16 IConvertible.ToInt16(... ToInt32 Method int IConvertible.ToInt32(Sy... ToInt64 Method long IConvertible.ToInt64(S... ToLower Method string ToLower(), string To... ToLowerInvariant Method string ToLowerInvariant() ToSByte Method sbyte IConvertible.ToSByte(... ToSingle Method float IConvertible.ToSingle... ToString Method string ToString(), string T... ToType Method System.Object IConvertible.... ToUInt16 Method uint16 IConvertible.ToUInt1... ToUInt32 Method uint32 IConvertible.ToUInt3... ToUInt64 Method uint64 IConvertible.ToUInt6... ToUpper Method string ToUpper(), string To... ToUpperInvariant Method string ToUpperInvariant() Trim Method string Trim(Params char[] t... TrimEnd Method string TrimEnd(Params char[... TrimStart Method string TrimStart(Params cha... Chars ParameterizedProperty char Chars(int index) {get;} Length Property int Length {get;}
You see that a string has a lot of methods, one property, and a special type of property called ParameterizedProperty
. Let's first use the Length
property. To use a property, type the object name or the name of the variable containing the object; then type a dot; finally, type the property name. For the string, you could use both of the following command lines:
PowerCLI C:\> "Learning PowerCLI".Length 17 PowerCLI C:\> $String.Length 17
You see that the Length
property contains the number of characters of the string "Learning PowerCLI"
, 17 in this case.
Property names in PowerShell are not case-sensitive, so you could type the following command and still get the same results:
PowerCLI C:\> $String.length 17
ParameterizedProperty
is a property that accepts a parameter value. The ParameterizedProperty
property's Chars
can be used to return the character at a specific position in the string. You have to specify the position, also called the index, as a parameter to Chars
. Indexes in PowerShell start with 0
. Therefore, to get the first character of the string, type the following command:
PowerCLI C:\> $String.Chars(0) L
To get the second character of the string, type the following command:
PowerCLI C:\> $String.Chars(1) e
You cannot use -1
to get the last character of the string as you can do with indexing in a PowerShell array. You have to calculate the last index yourself; this is calculated by subtracting 1 from the length of the string. Therefore, to get the last character of the string, you can type the following command:
PowerCLI C:\> $String.Chars($String.Length - 1) I
PowerShell has more types of properties, such as AliasProperty
, CodeProperty
, NoteProperty
, and ScriptProperty
.
AliasProperty
is an alias name for an existing propertyCodeProperty
is a property that maps to a static method on a .NET classNoteProperty
is a property that contains dataScriptProperty
is a property whose value is returned from executing a PowerShell scriptblock
Using methods
Using methods is as easy as using properties. You type the object name, or the name of a variable containing the object; then you type a dot; and, after the dot, you type the name of the method. For methods, you always have to use parentheses after the method name. For example, to modify a string to all uppercase letters, type in the following command:
PowerCLI C:\> $String.ToUpper() LEARNING POWERCLI
Some methods require parameters. For example, to find the index of the P
character in the string, you can use the following command:
PowerCLI C:\> $String.IndexOf('P') 9
The character P
is the tenth character in the "Learning PowerCLI"
string. But because indexes in PowerShell start with 0
and not 1
, the index of the P
character in the string is 9
and not 10
.
One very useful method is Replace
, which you can use to replace a character or a substring with another character or string or with nothing. For example you can replace all instances of the e
characters in the string with the u
character, as follows:
PowerCLI C:\> $String.Replace('e','u') Luarning PowurCLI
The characters in the method are case-sensitive. If you use an uppercase 'E'
, it won't find the letter and will replace nothing:
PowerCLI C:\> $String.Replace('E','U') Learning PowerCLI
You can also replace a substring with another string. Let's replace the word "PowerCLI"
with "VMware vSphere PowerCLI"
:
PowerCLI C:\> $String.Replace('PowerCLI','VMware vSphere PowerCLI') Learning VMware vSphere PowerCLI
There is also a –Replace
operator in PowerShell. You can use the -Replace
operator to carry out a regular expression-based text substitution on a string or a collection of strings as shown in the following command line:
PowerCLI C:\> $string -Replace 'e','u' Luarning PowurCLI
Although both have the same name, the string Replace
method and the –Replace
operator are two different things. There is no –ToUpper
operator; as you can see in the following example, this gives an error message:

You can use more than one method in the same command. Say you want to replace Learning
with Gaining
and that you want to remove the characters C
, L
, and I
from the end of the string using the TrimEnd
method. For this, you can use the following command:
PowerCLI C:\> $String.Replace('Learning','Gaining').TrimEnd('CLI') Gaining Power