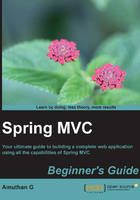
Time for action – understanding the web application context
You have received enough of an introduction on the web application context; now, tweak a little bit with the name and location of the web application context configuration file (DefaultServlet-servlet.xml
) and observe the effect. Perform the following steps:
- Rename the
DefaultServlet-servlet.xml
file toDispatcherServlet-servlet.xml
; you can findDefaultServlet-servlet.xml
under thesrc/main/webapp/WEB-INF/
directory. - Then, run your webstore project again and enter the URL,
http://localhost:8080/webstore/
; you will see an HTTP Status 500 error message on your web page and aFileNotFoundException
error in the stack trace as follows:java.io.FileNotFoundException: Could not open ServletContext resource [/WEB-INF/DefaultServlet-servlet.xml]
An error message displaying FileNotFoundException for DefaultServlet-servlet.xml
- To fix this error, change the name of
DefaultServlet
toDispatcherServlet
inweb.xml
; basically, after changing the name toDispatcherServlet
, your servlet configuration will look like the following in theweb.xml
file:<servlet> <servlet-name>DispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> </servlet> <servlet-mapping> <servlet-name>DispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping>
- Now, run your application and enter the URL,
http://localhost:8080/webstore/
; you will see the welcome message again. - Rename your
DispatcherServlet-servlet.xml
file toDispatcherServlet-context.xml
once more. - Next, create a directory structure
spring/webcontext/
under theWEB-INF
directory and move theDispatcherServlet-context.xml
file to thesrc/main/webapp/WEB-INF/spring/webcontext/
directory. - Then, run your application, and you will see an HTTP Status 500 error message on your web page again and a
FileNotFoundException
error message in the stack trace:java.io.FileNotFoundException: Could not open ServletContext resource [/WEB-INF/DispatcherServlet-servlet.xml]
- To fix this error, add the following tags within the
<servlet>
and</ servlet>
tags inweb.xml
as shown in the following code:<init-param> <param-name>contextConfigLocation</param-name> <param-value> /WEB-INF/spring/webcontext/DispatcherServlet-context.xml </param-value> </init-param>
- Now, run the application again and enter the URL,
http://localhost:8080/webstore/
; you will be able to see the welcome message again.
What just happened?
So, what we did first was renamed the DefaultServlet-servlet.xml
file to DispatcherServlet-servlet.xml
, and we got a FileNotFoundException
error at runtime, as follows:
java.io.FileNotFoundException: Could not open ServletContext resource [/WEB-INF/DefaultServlet-servlet.xml]
To fix the error, we changed our dispatcher servlet configuration, as follows, in the web.xml
file:
<servlet> <servlet-name>DispatcherServlet</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> </servlet> <servlet-mapping> <servlet-name>DispatcherServlet</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping>
We changed the servlet name to DispatcherServlet
in order to align with the web application context configuration file named DispatcherServlet-servlet.xml
. So, based on this exercise, we can learn that during the start-up of any Spring MVC project, the dispatcher servlet will look for a web application context configuration file of the pattern <Configured dispatcher Servlet Name>-servlet.xml
under the WEB-INF
directory. It is our responsibility to keep the web application context configuration file under the WEB-INF
directory with the right name. However, what if we wish to keep the file in some other directory?
Tip
One of the important things to be noted in <servlet-mapping>
is the value of the <url-pattern>/</url-pattern>
tag. By assigning /
as the URL pattern for the dispatcher servlet, we make DispatcherServlet
the default servlet for our web application. So, every web request coming to our web application will be handled by DispatcherServlet
.
For instance, in steps 5 and 6, we renamed the web application context configuration file and moved it to a completely new directory (src/main/webapp/WEB-INF/spring/webcontext/
). In that case, how did we fix the HTTP Status 500 error? The answer lies within a property called contextConfigLocation
. For the dispatcher servlet to locate the web context configuration file easily, we gave the location of this file to the dispatcher servlet through a property called contextConfigLocation
. That's why we added this property to the dispatcher servlet in step 8, as follows:
<servlet> <servlet-name>DispatcherServlet</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value> /WEB-INF/spring/webcontext/DispatcherServlet-context.xml </param-value> </init-param> </servlet>
Now, we are able to run our application without any problem. Okay, we played a lot with the web application context configuration file and learned that the dispatcher servlet should know about the web application context configuration file during the start-up of our project. So the next question is: why is the dispatcher servlet looking for this web context configuration file, and what is defined inside this file? Let's find out the answer, but before that, you may answer the following pop quiz questions to make sure you understand the concept of the web application context configuration.
Pop quiz – the web application context
Q1. If the contextConfigLocation
property was not configured in our dispatcher servlet configuration, under which location would Spring MVC look for the web application context configuration file?
- In the
WEB-INF
directory - In
WEB-INF/spring
- In
WEB-INF/spring/appServlet
Q2. If we do not want to provide contextConfigLocation
to the following dispatcher servlet configuration, how do we avoid the HTTP Status 500 error?
<servlet> <servlet-name>FrontController</servlet-name> <servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class> </servlet>
- By creating a context file called
FrontController-context.xml
in theWEB-INF
directory - By creating a file called
DispatcherServlet-context.xml
inWEB-INF
- By creating a file called
FrontController-servlet.xml
inWEB-INF