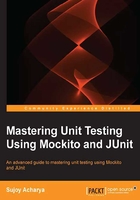
What this book covers
Chapter 1, JUnit 4 – a Total Recall, covers the unit testing concept, the JUnit 4 framework, the Eclipse setup, and advance features of JUnit 4. It covers the JUnit 4 framework briefly to get you up and running. We will discuss the concept surrounding JUnit essentials, annotations, assertion, the @RunWith annotation, and exception handling so that you have an adequate background on how JUnit 4 works. Advanced readers can skip to the next section. JUnit 4++ explores the advanced topics of JUnit 4 and deep dives into the following topics: parameterized test, matchers and assertThat, assumption, theory, timeout, categories, rules, test suites, and test order.
Chapter 2, Automating JUnit Tests, focuses on getting the reader quickly started with the Extreme Programming (XP) concept, Continuous Integration (CI), benefits of CI, and JUnit test automation using various tools such as Gradle, Maven, Ant, and Jenkins. By the end of this chapter, the reader will be able to write build scripts using Gradle, Maven, and Ant and configure Jenkins to execute the build scripts.
Chapter 3, Test Doubles, illustrates the concept of test doubles and explains various test double types, such as mock, fake, dummy, stub, and spy.
Chapter 4, Progressive Mockito, distills the Mockito framework to its main core and provides technical examples. No previous knowledge of mocking is necessary. By the end of this chapter, the reader will be able to use advanced features of the Mockito framework; start behavior-driven development using Mockito; and write readable, maintainable, and clean JUnit tests using Mockito.
Chapter 5, Exploring Code Coverage, unfolds the code coverage concept, code coverage tools, and provides step-by-step guidance to generate coverage reports using various build scripts. The following topics are covered: code coverage; branch and line coverage; coverage tools—Clover, Cobertura, EclEmma, and JaCoCo; measuring coverage using Eclipse plugins; and using Ant, Maven, and Gradle to generate reports. By the end of this chapter, the reader will be able to configure Eclipse plugins and build scripts to measure code coverage.
Chapter 6, Revealing Code Quality, explores the static code analysis and code quality improvement. By the end of this chapter, the reader will be able to configure the SONAR dashboard, set up Eclipse plugins, configure Sonar runner, and build scripts to analyze code quality using PMD, FindBugs, and Checkstyle.
Chapter 7, Unit Testing the Web Tier, deals with unit testing the web tier or presentation layer. It covers unit testing servlets, playing with Spring MVC, and working with the Model View Presenter pattern. By the end of this chapter, the reader will be able to unit test the web tier components and isolate the view components from the presentation logic.
Chapter 8, Playing with Data, covers the unit testing of the database layer. Topics such as separating concerns, unit testing the persistence logic, simplifying persistence with Spring, verifying the system integrity, and writing integration tests with Spring are explained. By the end of this chapter, the reader will be able to unit test the data access layer components in isolation from the database, write neat JDBC code using Spring, and write integration tests using the Spring API.
Chapter 9, Solving Test Puzzles, explains the importance of unit testing in greenfield and brownfield projects. Topics such as working with testing impediments, identifying constructor issues, realizing initialization issues, working with private methods, working with final methods, exploring static method issues, working with final classes, learning new concerns, exploring static variables and blocks, and test-driven development are covered. By the end of this chapter, the reader will be able to write unit tests for the legacy code; refactor the legacy code to improve the design of existing code; start writing simple, clean, and maintainable code following test-first and test-driven developments; and refactor code to improve code quality.
Chapter 10, Best Practices, focuses on JUnit guidelines and best practices for writing clean, readable, and maintainable JUnit test cases. It covers working with assertions, handling exceptions, and working with test smells. By the end of this chapter, the reader will be able to write clean and maintainable test cases.