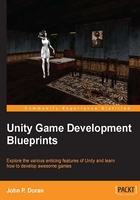
Project setup
At this point, I have assumed that you have a fresh installation of Unity, and have started it up. You need to perform the following steps:
- Open the previous project. Now, let's first create a new scene by navigating to File | New Scene. With the new scene created, save it by navigating to File | Save Scene. Name it
Main_Menu.unity
and save it inside yourScenes
folder. - Let's first grab the background from our previous level so that we do not need to create it once again. To do that, double-click on the scene you created in the first chapter. Left-click on the Background object in the Hierarchy view and navigate to Edit | Copy. Go back to your
Main_Menu
scene and paste it into the world by navigating to Edit | Paste:Unity has an inbuilt GUI functionality through the UnityGUI system that allows us to create interfaces through scripts.
Note
Unless you are using Unity 4.6 or later, Unity doesn't have an included visual GUI development system, but you can find tools on the Unity Asset Store that can be used to create your GUI in a What You See Is What You Get (WYSIWYG) fashion. Otherwise, you can also use Autodesk's popular Scaleform system, which allows you to create your GUIs in Adobe Flash for an additional cost.
This system uses a function called
OnGUI
, which is similar to theUpdate
function we used previously in the way that it gets called every frame that the component is enabled on a game object within the scene. All of your rendering of the GUI controls should be performed inside this function or in a function called inside of theOnGUI
function. - The first thing we're going to do is add a start button to our game. Right-click on the Scripts folder you created earlier, and then click on Create and select the C# Script label. Call this new script
MainMenuGUI
. Open MonoDevelop and use the following code:using UnityEngine; using System.Collections; public class MainMenuGUI : MonoBehaviour { public int buttonWidth = 100; public int buttonHeight = 30; void OnGUI() { //Get the center of our screen float buttonX = ( Screen.width - buttonWidth ) / 2.0f; float buttonY = ( Screen.height - buttonHeight ) / 2.0f; //Show button on the screen and check if clicked if ( GUI.Button( new Rect( buttonX, buttonY, buttonWidth, buttonHeight ), "Start Game" ) ) { // If button clicked, load the game level Application.LoadLevel("Chapter_1"); } // Add game's title to the screen, above our button GUI.Label( new Rect(buttonX + 2.5f , buttonY - 50, 110.0f, 20.0f), "Twinstick Shooter" ); } }
What this code basically does is place a title and button on our screen. Whenever we click on the button, the next level will be loaded. Now, this code may look quite different from what we've done before, so I will explain it after we see exactly what this code does.
- After saving the code, attach the
MainMenuGUI
script to the Main Camera object by dragging the script onto the object in the Hierarchy view. Once you complete that, save everything and start up the game! The following screenshot shows what we've created:At this point, you should have a very simple main menu screen. Now when we click on the Start Game button, we will move on to our game!
Tip
If you are not able to load the game level, make sure that your Scene from the last chapter is named
Chapter_1
and it is included in the Build Settings.
The anatomy of a GUI control
The most important concepts to grasp first are the GUI.Button
and GUI.Label
functions. Both of these functions are what we refer to as a GUI control, and there are many others which we will be using in the future. So, to clear up any confusion, let's talk about them now.
Creating any GUI control consists of the following:
ControlType(Position, Content)
The parts of the preceding line of code are explained in the following sections.
ControlType
The ControlType
function is a function that exists in Unity's GUI class, and is the name of the element that you want to create in the world. In the preceding code, we used GUI.Button
and GUI.Label
, but there are many more.
Note
For more information on all the different kinds of controls, there are additional definitions for the functions. You can check them out at https://docs.unity3d.com/Documentation/Components/gui-Controls.html.
Position
The Position
parameter is where you want to place the object in the screen space. In the previous chapter, we talked about viewport and screen space (pixel space). When working with the GUI class like we are in this example, we have to manually position each element that we place on the screen based on the screen space in pixels. To do that, we want to know the position (x, y) that we want to place the object at, and the size (width, height) of that object. These are the four parameters of the Rect
structure, which itself is the first parameter that we provide to many of the GUI class functions.
Since we want this code to work on as many projects as possible, I don't want to hardcode what the middle of the screen is. Thankfully, the Screen.width
and Screen.height
properties can be used to let me know the current dimensions of the screen in pixels, no matter what screen size the game is on.
The first thing I need to know is how large I want my displayed objects to be. The constructor of the Rect
class takes in four floats, so I need to use floating point numbers (add .0f
to whole number values to use them as floats).
For my button, I'm creating two variables, buttonWidth
and buttonHeight
, to fill in values for the third and fourth parameters of the Rect
variable.
By setting the buttonX
and buttonY
values inside the OnGUI
function, if at any time during the game we change the resolution of the game or port our game to other places, the GUI will work without any modifications, which is pretty great. For the title of our screen, I'll just put in some values to show that we do not need to create variables for this.
The X and the Y position set the top-left of our object, which is great if we're putting something on the top-left. However, if we just set the middle of the screen, it will be a tad offset. So, we'll need to move our position to fit the size of our buttons as well.
Content
The second and final argument for the control is the actual content that we want to display with the ControlType we are using. Right now, we're just passing in a string to display, but we can also display images and other content as well, including other controls. We will talk about other pieces of content that we can add in later.
GUI.Button
One of the most common UI elements is the Button
control. This function is used to render a clickable object. If you look at the code from the MainMenuGUI
script, you'll notice that the button is cased inside of an if
statement. This is because if the button is clicked on, then the function will return true
when the button is released. If this is true, we will load the level we created in the previous chapter.
GUI.Label
The Label
control is used to display static data on the screen that the player will not be able to interact with. This is quite similar to the GUIText
objects that we created in the previous chapter. Labels can contain text, textures, or both. In our case, we are just displaying some text.