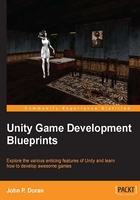
Adding in sound effects/music
Another thing that we can do to give the project a little more polish is add in sound effects and background music. Let's do that now. Perform the following steps:
- Select your enemy prefab, and add an Audio Source component to it by selecting Component | Audio | Audio Source. An audio source lets the audio listener into Main Camera knowing that this is an object that can play sounds. Once you create the Audio Source, open the 3D Sound Settings and change the Min Distance of the Volume Rolloff to
10
. Unity will attempt to alter the volume and pan sounds to make the project sound nicer, but for this project, it just makes everything much softer, so we're going to undo the effect unless it's far away. - After this, let's go into our
EnemyBehaviour
script! As usual, we'll need to add in a new variable for us to use to play whenever we're hit:// What sound to play when we're hit public AudioClip hitSound;
- Next, go into the
CollisionEnter2D
function. After theDestroy(theCollision.gameObject)
line, add the following code:// Plays a sound from this object's AudioSource audio.PlayOneShot(hitSound);
Note
For more information about the
PlayOneShot
function, check out http://docs.unity3d.com/ScriptReference/AudioSource.PlayOneShot.html.For more information on the Audio Source component (audio), check out http://docs.unity3d.com/ScriptReference/AudioSource.html.
Now, we need some actual sounds to play. I've set aside a folder of assets for you in the Example Code
folder, so drag-and-drop the Sounds
folder into your project's Assets
folder:
- Back in the inspector for our enemy, let's set the
Hit Sound
variable in theEnemyBehaviour
script to the hit sound that we've imported by using drag-and-drop. Now if we play the game, when we hit an enemy, the sound will be played! Now, let's have a sound if we destroy the enemy! - Go to the Explosion prefab, and add an Audio Source component in the same way we did in step 1. Once you create the audio source, open up the 3D Sound Settings, and change the Min Distance of the Volume Rolloff to
10
. After this, set the Audio Clip property in the component to theexplode
sound. - Now, going back to our
EnemyBehaviour
script, go to the line after we instantiate theexploder
object, and add the following line before we destroy the exploder:exploder.audio.Play();
Now, if you play the game, hitting the object will play one sound, and when the object is destroyed, the explosion will play a sound. Because the sound is in the Audio Clip property, we can just call the
Play
function. However, if you want an object to play multiple sounds, it's better to have separateAudioClip
variables just as we did withEnemyBehaviour
. - Finally, I want to play a sound whenever we fire a shot. To do that, let's go to
playerShip
and add an audio source. Once you create the audio source, open 3D Sound Settings, and change the Min Distance of Volume Rolloff to10
. - Next, go into
PlayerBehaviour
, and add in a new variable, as follows:// What sound to play when we're shooting public AudioClip shootSound;
- After this, whenever we shoot a bullet, let's play the new sound at the beginning of the
ShootLasers
function:audio.PlayOneShot(shootSound);
- Coming back to Inspector, set the Shoot Sound property in the
PlayerBehaviour
component to theshoot
sound effect. - Finally, let's add in our background music. Go to your Main Camera object in Hierarchy. Add an Audio Source component. There's no need to set Min Distance in this case, because this object is where the audio listener is. Change Audio Clip to bgm, check the Loop option, and set the Volume to
.25
.Note
The background music is provided for this project by Stratkat (Kyle Smith). If you are interested in more of his work, check out his website at http://daydreamanatomy.bandcamp.com/.
- Save everything, and run the game!
While we won't see any changes at this point for those of you actually running the game, you'll notice quite a change when the game is started. It's already feeling much more like a game.
Note
If you don't want to deal with the 3D settings, you can also select the sound files and uncheck the 3D sound option, but this will give you less control.