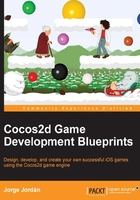
2-star challenge – create explosions
Now that we are able to detect when a UFO is destroyed, it would be more realistic to make them explode, and I think you're ready to develop this by yourself.
Create one explosion whenever a spaceship is destroyed by using the file explosion.png
that you will find in the Resources
folder.
The solution
You will need to go back a few pages to achieve this challenge because in this case, we're going to create a CCParticleSystemModeRadius
particle.
First of all, add explosion.png
to the project and then add the following lines inside the method detectCollisions
, after [_ufosToRemove addObject:ufo];
:
CCParticleExplosion *explosion = [CCParticleExplosion node]; explosion.texture = [CCTexture textureWithFile:@"explosion.png"]; explosion.emitterMode = CCParticleSystemModeRadius; explosion.startSize = 100.0; explosion.startRadius = 20.0; explosion.endSize = 1.0; explosion.endRadius = 100.0; explosion.totalParticles = 200; explosion.life = 2.0; explosion.lifeVar = 0.0; explosion.emissionRate = explosion.totalParticles/explosion.life; explosion.position = ufo.position; [self addChild:explosion z:1];
Now you will have an impressive explosion!

The attack of the aliens
Aliens have invaded the planet Earth to kill humanity and they have come fully armed with high technology red laser beams. In this section, we're going to implement their attack.
First of all, we need to add the image to create the red laser beams, so follow the next steps:
- Right-click on the Resources group and select Add Files to "ExplosionsAndUFOs"….
- In the
Resources
folder, you will findlaser_red.png
, so select it and click on Add.
Then we're going to proceed as in the green laser's case. Let's declare an array and a maximum number of red lasers. Add these lines after NSMutableArray *_ufosToRemove;
:
// Declare array of red lasers NSMutableArray *_arrayLaserRed; // Max number of lasers in scene int _numLaserRed;
Initialize them by adding the following in the init
method, before the return self;
statement:
// Initialize max number of red lasers _numLaserRed = 15; // Initialize the array of red lasers _arrayLaserRed = [NSMutableArray arrayWithCapacity:_numLaserRed];
For now, we are doing things we already know; we just initialized the maximum number of red lasers to be 15
and then initialized the red lasers array.
As we want the spaceships to shoot from time to time, we're going to schedule a method for this purpose. Include this line at the bottom of the init
method, before return self;
:
// Shoot red lasers [self schedule:@selector(shootRedLaser:) interval:2.0f];
Implement it by adding the following block of code:
// Shoot red laser beams -(void)shootRedLaser:(CCTime) delta { CCSprite *laserRed; // Shoot lasers if there are UFOs in scene and hasn't reached the max number of red lasers if ([_arrayUFOs count] > 0 && [_arrayLaserRed count] < _numLaserRed){ // For each UFO on the scene for(UFO *ufo in _arrayUFOs) { // Create red laser sprite laserRed = [CCSprite spriteWithImageNamed:@"laser_red.png"]; // Set red laser position laserRed.position = CGPointMake(ufo.position.x, ufo.position.y - ufo.contentSize.height / 2); // Add laser to array of lasers [_arrayLaserRed addObject:laserRed]; // Add laser to scene [self addChild:laserRed]; // Declare laser speed float laserSpeed = 600.0; // Calculate laser's final position CGPoint nextPosition = CGPointMake(laserRed.position.x, -laserRed.contentSize.height / 2); // Calculate duration float laserDuration = ccpDistance(nextPosition, laserRed.position) / laserSpeed; // Move red laser sprite out of the screen CCActionMoveTo *actionLaserRed = [CCActionMoveTo actionWithDuration:laserDuration position:nextPosition]; // Action to be executed when the red laser reaches its final position CCActionCallBlock *callDidMove = [CCActionCallBlock actionWithBlock:^{ // Remove laser from array and scene [_arrayLaserRed removeObject:laserRed]; [self removeChild:laserRed]; }]; CCActionSequence *sequenceLaserRed = [CCActionSequence actionWithArray:@[actionLaserRed, callDidMove]]; // Run action sequence [laserRed runAction:sequenceLaserRed]; } } }
This code is pretty easy to understand. The first thing we do is check whether there is a UFO in the scene and whether the number of existing red lasers in the scene is lower than the maximum number of red lasers allowed. If these conditions are met, then we iterate the UFO
objects array and perform two main actions.
First, we create a new laser sprite with the image we just added to the project and set its initial position on the bottom-center point of the UFO and add it to the scene.
Second, we set up the movement action for the spaceship. We calculate the duration of the laser displacement to the bottom of the screen at a constant speed of 600 and then we use this value to create a CCActionMoveTo
instance. We create a CCActionCallBlock
instance where we will remove the laser from the scene and from the array of red lasers, and then we execute the actions sequence.
Run the project and take a look at the results!

We just need to implement the collisions between red lasers and Dr. Fringe so we're going to proceed in a similar manner as we did with green lasers and UFO objects.
First, declare the auxiliary array we will use to remove collided red lasers by adding the following line just after int _numLaserRed;
:
// Array of removable laser beams NSMutableArray *_lasersRedToRemove;
Initialize it in the init
method by adding this line after _ufosToRemove = [NSMutableArray array];
:
_lasersRedToRemove = [NSMutableArray array];
Then, in detectCollisions
, add the following block of code at the end of the method:
CCSprite *laserRed; // For each red laser beam shot for (laserRed in _arrayLaserRed){ // Detect laserRed-scientist collision if (CGRectIntersectsRect(_scientist.boundingBox, laserRed.boundingBox)) { // Stopping laser beam actions [laserRed stopAllActions]; // Adding the object to the removable objects array [_lasersRedToRemove addObject:laserRed]; // Remove the laser from the scene [self removeChild:laserRed]; CCLOG(@"RED LASER COLLISION"); } } // Remove objects from array [_arrayLaserRed removeObjectsInArray:_lasersRedToRemove];
This code is very similar to the green laser-UFO case. We iterate the red lasers array and try to find out whether some of them collide with our scientist. In this case, we stop all red laser actions, add it to the removable objects array, and remove the child from the scene. Once the loop has finished, we can remove the collided lasers from their array.