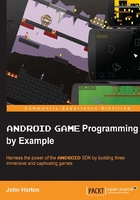
Building the home screen
Since we have done all the planning and preparation, we can get started with the code.
Note
Downloading the example code
You can download the example code files from your account at http://www.packtpub.com for all the Packt Publishing books you have purchased. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
To use the code files, you will still need to create an Android Studio project. In addition, you will need to change the package name in the very first line of code of each of the JAVA files. Change the package name to match the package name of the project you created. Finally, you will need to make sure that any assets such as images or sound files are placed into the appropriate folder in the project. All the required assets for each project are supplied in the download bundle.
Creating the project
Fire up Android Studio and create a new project by following these steps. All the files to get the project to where we will be, by the end of this chapter, are in the download bundle in the Chapter2
folder.
- On the Welcome to Android Studio dialog, click on Start a new Android Studio project.
- In the Create New Project window shown next, we need to enter some basic information about our app. These bits of information will be used by Android Studio to determine the package name.
Note
In the following image, you can see the Edit link where you can customize the package name if required.
- If you will be copy/pasting the supplied code into your project, then use
C1 Tappy Defender
for the Application name field andgamecodeschool.com
in the Company Domain field as shown in the following screenshot: - Click on the Next button when you're ready. When asked to select the form factors, your app will run on, we can accept the default settings (Phone and Tablet). So click on Next again.
- On the Add an activity to mobile dialog, just click on Blank Activity followed by the Next button.
- On the Customize the Activity dialog, again we can accept the default settings because
MainActivity
seems like a good name for our main Activity. So click on the Finish button.
What we did
Android Studio has built the project and created a number of files, most of which we will see and edit during the course of building this game. As mentioned earlier, even if you are just copying and pasting the code, you need to go through this step because Android Studio is doing things behind the scenes to make our project work.
Building the home screen UI
The first and simplest part of our Tappy Defender game is the home screen. All we need is a neat picture with a scene about the game, a high score, and a button to start the game. The finished home screen will look a bit like this:

When we built the project, Android Studio opens up two files ready for us to edit. You can see them as tabs in the following Android Studio UI designer. The files (and tabs) are MainActivity.java
and activity_main.xml
:

The MainActivity.java
file is the entry point to our game, and we will see this in more detail soon. The activity_main.xml
file is the UI layout that our home screen will use. Now, we can go ahead and edit the activity_main.xml
file, so it actually looks like our home screen should.
- First of all, your game will be played with the Android device in landscape mode. If we change our UI preview window to landscape, we will see your progress more accurately. Look for the button shown in the next image. It is just preceding the UI preview:
- Click on the button shown in the preceding screenshot, and your UI preview will switch to landscape like this:
- Make sure
activity_main.xml
is open by clicking on its tab. - Now, we will set in a background image. You can use your own or mine from
Chapter2/drawable/background.jpg
in the download bundle. Add your chosen image to thedrawable
folder of the project in Android Studio. - In the Properties window of the UI designer, find and click on the background property as shown in the next image:
- Also, in the previous image the button labelled ... is outlined. It is just to the right of the background property. Click on that ... button and browse to and select the background image file that you will be using.
- Next, we need a TextView widget that we will use to display the high score. Note that there is already a TextView widget on the layout. It says Hello World. You will modify this and use it for our high score. Left click on it and drag the TextView to where you want it. You can copy me if you intend using the supplied background or put it where it looks best with your background.
- Next, in the Properties window, find and click on the id property. Enter
textHighScore
. Type it exactly as shown because when we write some Java code in a later tutorial, we will refer to this ID in order to manipulate it, to show the player's fastest time. - You can also edit the text property to say
High Score: 99999
or similar so that the TextView looks the part. However, this isn't necessary because your Java code will take care of this later. - Now, we will drag a button from the widget palette as shown in the following screenshot:
- Drag it to where it looks good on your background. You can copy me if using the supplied background or put it where it looks best with your background.
What we did
We now have a cool background with neatly arranged widgets (a TextView and a Button) for your home screen. We can add functionality via Java code to the Button widget next. Revisit the TextView for the player's high score in Chapter 4, Tappy Defender – Going Home. The important point is that both the widgets have been assigned a unique ID that we can use to reference and manipulate in your Java code.
Coding the functionality
Now, we have a simple layout for our game home screen. Now, we need to add the functionality that will allow the player to click on the Play button to start the game.
Click on the tab for the MainActivity.java
file. The code that was automatically generated for us is not exactly what we need. Therefore, we will start again as it is simpler and quicker than tinkering with what is already there.
Delete the entire contents of the MainActivity.java
file except the package name and enter the following code in it. Of course, your package name may be different.
package com.gamecodeschool.c1tappydefender; import android.app.Activity; import android.os.Bundle; public class MainActivity extends Activity{ // This is the entry point to our game @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); //Here we set our UI layout as the view setContentView(R.layout.activity_main); } }
The mentioned code is the current contents of our main MainActivity
class and the entry point of our game, the onCreate
method. The line of code that begins with setContentView...
is the line that loads our UI layout from activity_main.xml
to the players screen. We can run the game now and see our home screen, but let's make some more progress, following which we will look at how we run the game on a real device at the end of the chapter.
Now, let's handle the Play button on our home screen. Add the two highlighted lines of the following code into the onCreate
method just after the call to setContentView()
. The first new line creates a new Button
object and gets a reference to Button
in our UI layout. The second line is the code that listens for clicks on the button.
//Here we set our UI layout as the view setContentView(R.layout.activity_main); // Get a reference to the button in our layout final Button buttonPlay = (Button)findViewById(R.id.buttonPlay); // Listen for clicks buttonPlay.setOnClickListener(this);
Note that we have a few errors in our code. We can resolve these errors by holding down the Alt keyboard key and then pressing Enter. This will add an import directive for the Button
class.
We still have one error. We need to implement an interface so that our code listens to the button clicks. Modify the MainActivity
class declaration as highlighted:
public class MainActivity extends Activity
implements View.OnClickListener{
When we implement the onClickListener
interface, we must also implement the onClick
method. This is where we will handle what happens when a button is clicked. We can automatically generate the onClick
method by right-clicking somewhere after the onCreate
method, but within the MainActivity
class, and navigating to Generate | Implement methods | onClick(v:View):void. Or just add the given code.
We also need to have Android Studio add another import directive for Android.view.View
. Use the Alt | Enter keyboard combination again.
We can now scroll to near the bottom of the MainActivity
class and see that Android Studio has implemented an empty onClick
method for us. We should have no errors in your code at this point. Here is the onClick
method:
@Override public void onClick(View v) { //Our code goes here }
As we only have one Button
object and one listener, we can safely assume that any clicks on our home screen are the player pressing our Play button.
Android uses the Intent
class to switch between activities. As we need to go to a new activity when the Play button is clicked, we will create a new Intent
object and pass in the name of our future Activity
class, GameActivity
to its constructor. We can then use the Intent
object to switch activities. Add the following code to the body of the onClick
method:
// must be the Play button. // Create a new Intent object Intent i = new Intent(this, GameActivity.class); // Start our GameActivity class via the Intent startActivity(i); // Now shut this activity down finish();
Once again, we have errors in our code because we need to generate a new import directive, this time for the Intent
class so use the Alt | Enter keyboard combination again. We still have one error in our code. This is because our GameActivity
class does not exist yet. We will now solve this problem.
Creating GameActivity
We have seen that when the player clicks on the Play button, main activity will close and game activity will begin. Therefore, we need to create a new activity called GameActivity
that will be were your game actually executes.
- From the main menu, navigate to File | New | Activity | Blank Activity.
- In the Customize the Activity dialog, change the Activity Name field to
GameActivity
. - We can accept all the other default settings from this dialog, so click on Finish.
- As we did with your
MainActivity
class, we will code this class from scratch. Therefore, delete the entire code content fromGameActivity.java
.
What we did
Android Studio has generated two more files for us and done some work behind the scenes that we will investigate soon. The new files are GameActivity.java
and activity_game.xml
. They are both automatically opened for us in two new tabs, in the same place as the other tabs above the UI designer.
We will never need activity_game.xml
because we will build a dynamically generated game view, not a static UI. Feel free to close that now or just ignore it. We will come back to the GameActivity.java
file, when we start to code our game for real, later in the chapter in the Coding the game loop section.
Configuring the AndroidManifest.xml file
We briefly mentioned that when we create a new project or a new activity, Android Studio does more than just creating two files for us. This is why we create new projects/activities the way we do.
One of the things going on behind the scenes is the creation and modification of the AndroidManifest.xml
file in the manifests
directory.
This file is required for our app to work. Also, it needs to be edited to make our app work the way we want it to. Android Studio has automatically configured the basics for us, but we will now do two more things to this file.
By editing the AndroidManifest.xml
file, we will force both of our activities to run with a full screen, and we will also lock them to a landscape layout. Let's make these changes here:
- Open the
manifests
folder now, and double click on theAndroidManifest.xml
file to open it in the code editor. - In the
AndroidManifest.xml
file, find the following line of code:android:name=".MainActivity"
- Immediately following it, type or copy and paste these two lines to make
MainActivity
run full screen and lock it in the landscape orientation:android:theme="@android:style/Theme.NoTitleBar.Fullscreen" android:screenOrientation="landscape"
- In the
AndroidManifest.xml
file, find the following line of code:android:name=".GameActivity"
- Immediately following it, type or copy and paste these two lines to make
GameActivity
run full screen and lock it in the landscape orientation:android:theme="@android:style/Theme.NoTitleBar.Fullscreen" android:screenOrientation="landscape"
What we did
We have now configured both activities from our game to be full screen. This presents a much more pleasing appearance to our player. In addition, we have disabled the player's ability to affect our game by rotating their Android device.