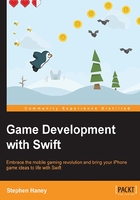
上QQ阅读APP看书,第一时间看更新
Putting it all together
First, we learned how to use actions to move, scale, and rotate our sprites. Then, we explored animating through multiple frames, bringing our sprite to life. Let us now combine these techniques to fly our bee back and forth across the screen, flipping the texture at each turn.
Add this code at the bottom of the didMoveToView
function, beneath the bee.runAction(beeAction)
line:
// Set up new actions to move our bee back and forth: let pathLeft = SKAction.moveByX(-200, y: -10, duration: 2) let pathRight = SKAction.moveByX(200, y: 10, duration: 2) // These two scaleXTo actions flip the texture back and forth // We will use these to turn the bee to face left and right let flipTextureNegative = SKAction.scaleXTo(-1, duration: 0) let flipTexturePositive = SKAction.scaleXTo(1, duration: 0) // Combine actions into a cohesive flight sequence for our bee let flightOfTheBee = SKAction.sequence([pathLeft, flipTextureNegative, pathRight, flipTexturePositive]) // Last, create a looping action that will repeat forever let neverEndingFlight = SKAction.repeatActionForever(flightOfTheBee) // Tell our bee to run the flight path, and away it goes! bee.runAction(neverEndingFlight)
Run the project. You will see the bee flying back and forth, flapping its wings. You have officially learned the fundamentals of animation in SpriteKit! We will build on this knowledge to create a rich, animated game world for our players.