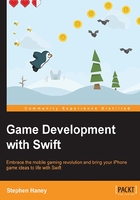
Adding textures and game art
You may want to take a screenshot of your blue box for your own enjoyment later. I absolutely love reminiscing over old screenshots of my finished games when they were nothing more than simple colored blocks sliding around the screen. Now it is time to move past that stage and attach some fun artwork to our sprite.
Downloading the free assets
I am providing a downloadable pack for all of the art assets I use in this book. I recommend you use these assets so you will have everything you need for our demo game. Alternatively, you are certainly free to create your own art for your game if you prefer.
These assets come from an outstanding public domain asset pack from Kenney Game Studio. I am providing a small subset of the asset pack that we will use in our game. Download the game art from this URL:
http://www.thinkingswiftly.com/game-development-with-swift/assets
More exceptional art
If you like the art, you can download over 16,000 game assets in the same style for a small donation at http://kenney.itch.io/kenney-donation. I do not have an affiliation with Kenney; I just find it admirable that he has released so much public domain artwork for indie game developers.
As CC0 assets, you can copy, modify, and distribute the art, even for commercial purposes, all without asking permission. You can read the full license here:
https://creativecommons.org/publicdomain/zero/1.0/
Drawing your first textured sprite
Let us use some of the graphics you just downloaded. We will start by creating a bee sprite. We will add the bee texture to our project, load the image onto a SKSpriteNode
class, and then size the node for optimum sharpness on retina screens.
Adding the bee image to your project
We need to add the image files to our Xcode project before we can use them in the game. Once we add the images, we can reference them by name in our code; SpriteKit is smart enough to find and implement the graphics. Follow these steps to add the bee image to the project:
- Right-click on your project in the project navigator and click on Add Files to "Pierre Penguin Escapes the Antarctic" (or the name of your game). Refer to this screenshot to find the correct menu item:
- Browse to the asset pack you downloaded and locate the
bee.png
image inside theEnemies
folder. - Check Copy items if needed, then click Add.
You should now see bee.png
in your project navigator.
Loading images with SKSpriteNode
It is quite easy to draw images to the screen with SKSpriteNode
. Start by clearing out all of the code we wrote for the blue square inside the didMoveToView
function in GameScene.swift
. Replace didMoveToView
with this code:
override func didMoveToView(view: SKView) { // set the scene's background to a nice sky blue // Note: UIColor uses a scale from 0 to 1 for its colors self.backgroundColor = UIColor(red: 0.4, green: 0.6, blue: 0.95, alpha: 1.0); // create our bee sprite node let bee = SKSpriteNode(imageNamed: "bee.png") // size our bee node bee.size = CGSize(width: 100, height: 100) // position our bee node bee.position = CGPoint(x: 250, y: 250) // attach our bee to the scene's node tree self.addChild(bee) }
Run the project and witness our glorious bee – great work!

Designing for retina
You may notice that our bee image is quite blurry. To take advantage of retina screens, assets need to be twice the pixel dimensions of their node's size property (for most retina screens), or three times the node size for the iPhone 6 Plus. Ignore the height for a moment; our bee node is 100 points wide but the PNG file is only 56 pixels wide. The PNG file needs to be 300 pixels wide to look sharp on the iPhone 6 Plus, or 200 pixels wide to look sharp on 2x retina devices.
SpriteKit will automatically resize textures to fit their nodes, so one approach is to create a giant texture at the highest retina resolution (three times the node size) and let SpriteKit resize the texture down for lower density screens. However, there is a considerable performance penalty, and older devices can even run out of memory and crash from the huge textures.
The ideal asset approach
These double- and triple-sized retina assets can be confusing to new iOS developers. To solve this issue, Xcode normally lets you provide three image files for each texture. For example, our bee node is currently 100 points wide and 100 points tall. In a perfect world, you would provide the following images to Xcode:
Bee.png
(100 pixels by 100 pixels)Bee@2x.png
(200 pixels by 200 pixels)Bee@3x.png
(300 pixels by 300 pixels)
However, there is currently an issue that prevents 3x textures from working correctly with texture atlases. Texture atlases group textures together and increase rendering performance dramatically (we will implement our first texture atlas in the next section). I hope that Apple will upgrade texture atlases to support 3x textures in Swift 2. For now, we need to choose between texture atlases and 3x assets for the iPhone 6 Plus.
My solution for now
In my opinion, texture atlases and their performance benefits are key features of SpriteKit. I will continue using texture atlases, thus serving 2x images to the iPhone 6 Plus (which still looks fairly sharp). This means that we will not be using any 3x assets in this book.
Further simplifying matters, Swift only runs on iOS7 and higher. The only non-retina devices that run iOS7 are the aging iPad 2 and iPad mini 1st generation. If these older devices are important for your finished games, you should create both standard and 2x images for your games. Otherwise, you can safely ignore non-retina assets with Swift.
Note
This means that we will only use double-sized images in this book. The images in the downloadable asset bundle forgo the 2x suffix, since we are only using this size. Once Apple updates texture atlases to use 3x assets, I recommend that you switch to the methodology outlined in The ideal asset approach section for your games.
Hands-on with retina in SpriteKit
Our bee image illustrates how this all works:
- Because we set an explicit node size, SpriteKit automatically resizes the bee texture to fit our 100-point wide, 100-point tall sized node. This automatic size-to-fit is very handy, but notice that we have actually slightly distorted the aspect ratio of the image.
- If we do not set an explicit size, SpriteKit sizes the node (in points) to the match texture's dimensions (in pixels). Go ahead and delete the line that sets the size for our bee node and re-run the project. SpriteKit maintains the aspect ratio automatically, but the smaller bee is still fuzzy. That is because our new node is 56 points by 48 points, matching our PNG file's pixel dimensions of 56 pixels by 48 pixels . . . yet our PNG file needs to be 112 pixels by 96 pixels for a sharp image at this node size on 2x retina screens.
- We want a smaller bee anyway, so we will resize the node rather than generate larger artwork in this case. Set the
size
property of your bee node, in points, to half the size of the texture's pixel resolution:// size our bee in points: bee.size = CGSize(width: 28, height: 24)
Run the project and you will see a smaller, crystal sharp bee, as in this screenshot:

Great! The important concept here is to design your art files at twice the pixel resolution of your node point sizes to take advantage of 2x retina screens, or three times the point sizes to take full advantage of the iPhone 6 Plus. Now we will look at organizing and animating multiple sprite frames.