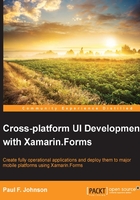
What is Xamarin Forms?
Xamarin Forms is known as a user interface abstraction library. It operates as a Portable Class Library (PCL), sitting on the target platforms and feeding in the UI elements that the application needs. It is as good as it sounds! Application user interfaces can be quickly constructed as a large amount of any other code can be shared.
Being an abstraction layer, only those elements with an analogous element on all of the target platforms are covered. For example, at the time of writing, there is not a single checkbox in forms and graphics on tab pages, for Android gives nothing.
This library is also part of a PCL. The PCL itself only supports a subset of the standard .NET library classes, namely those that are supported on all platforms. This leads to quite a few issues, such as the likes of the File
class within System
is simply not there as there is no guarantee that the targets will have the same class. This seems daft when you consider that everything supports files. This is both true and false. Mobiles and desktops have a file system, but embedded devices or devices with a sole project that needs no form of storage may have a custom build of the .NET libraries to exclude the classes not required (though they can be implemented using Wrapper classes).
Xamarin Forms are split into four main areas:
- Pages
- Layouts
- Views
- Cells
Pages
A page occupies the full screen in most cases. A page acts the same as Page
on a Windows Phone and UIViewController
on iOS. It is important to note that on Android they are more like a Resource.Layout
and are certainly not an Activity
.
There are five types of pages, given as follows:

Layouts
A layout is a container that can hold other layouts or views. There are seven different layouts, given as follows:

Views
A view is the likes of a Button
, ActivityIndicator
, or Label
. The 19 different types of views are given as follows:

Cells
A cell can be considered as a specialized view that describes how each item within a TableView
or ListView
is drawn. There are four cell types, given as follows:

So, how does this all work?
What follows gives you an idea on how Forms works. It is not the full story; that would take a great deal of time. What follows should suffice.
Before you consider how Xamarin Forms works, you need to consider how any application works, or its life cycle. The following screenshot shows the life cycles of Android, iOS, and Windows Phones applications.

http://i.stack.imgur.com/Jn6MZ.png
Tip
Downloading the example code
You can download the example code files for all Packt books you have purchased from your account at http://www.packtpub.com. If you purchased this book elsewhere, you can visit http://www.packtpub.com/support and register to have the files e-mailed directly to you.
The life cycle for a Windows Phone is not quite the same, as is shown in the following diagram:

http://edglogowski.azurewebsites.net/wp-content/uploads/2013/10/WinPhoAppLifecycle_thumb.png
In effect, they follow the process:
- Start
- Make the UI visible
- Handle events
- End
As all three follow the same routes and are using the same language, it should be possible to create a library that handles all these facilities. The problem is linking it in. Do you create three libraries with the same method names, but map down to different UI element names and call those from within the PCL?
Essentially, that is what is done (there is a lot more to it than that in reality). The library is instantiated on the platform with the UI being constructed from the code in the PCL. The PCL tells the platform, "I want a button in the middle of the view". Android uses a vertical layout, adds a button with the linear layout gravity set as center. The PCL then says, "the click event calls a method inside of the PCL, so redirect to that when it's called", and so on.
The life cycle is also used (though in a simpler way) as follows:
OnStart()
: This method is called when the app startsOnSleep()
: This method is called when the app goes into the background or when the app terminates naturally (not a crash)OnResume()
: This method is called when the app comes back from the background
A static Dictionary<string, object>
called Properties is also available
for storing information within the application(accessed through Application.Current.Properties
).
It follows all the usual rules for dictionaries. The Properties
dictionary is a persistent type (in other words, the dictionary stays even in the OnSleep()
state or when restarted. This is very useful to prevent the loss of information even when recovering from a crash.