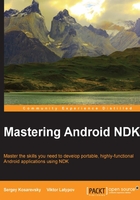
What this book covers
Chapter 1, Using Command-line Tools, shows you how to install and configure the essential tools for Android native development using the command line and how to write basic Android application configuration files manually from scratch without falling back on the graphical IDEs.
Chapter 2, Native Libraries, shows you how to build popular C/C++ libraries and link them against your applications using the Android NDK. These libraries are the building blocks to implement feature-rich applications with images, videos, sounds, and networking entirely in C++. We will show you how to compile libraries and, of course, give some examples and hints on how to start using them. Some of the libraries are discussed in greater detail in the subsequent chapters.
Chapter 3, Networking, focuses on how to deal with network-related functionality from the native C/C++ code. Networking tasks are asynchronous by nature and unpredictable in terms of timing. Even when the underlying connection is established using the TCP protocol, there is no guarantee on the delivery time, and nothing prevents the applications from freezing while waiting for the data. We will take a closer look at implementing basic asynchronous mechanisms in a portable way.
Chapter 4, Organizing a Virtual Filesystem, implements low-level abstractions to deal with the OS-independent access to files and filesystems. We will show how to implement portable and transparent access to the Android assets packed inside the .apk
files without falling back on any built-in APIs. This approach is necessary when building multiplatform applications that are debuggable in a desktop environment.
Chapter 5, Cross-platform Audio Streaming, implements a truly portable audio subsystem for Android and desktop PCs based on the OpenAL library. The code makes use of the multithreading material from Chapter 3, Networking.
Chapter 6, OpenGL ES 3.1 and Cross-platform Rendering, focuses on how to implement an abstraction layer on top of OpenGL 4 and OpenGL ES 3 to make our C++ graphics applications runnable on Android and desktop machines.
Chapter 7, Cross-platform UI and Input System, details the description of a mechanism to render geometric primitives and Unicode text. The second part of the chapter describes a multi-page graphical user interface suitable for being the cornerstone for building the interfaces of multiplatform applications. This chapter concludes with an SDL application, which demonstrates the capabilities of our UI system in action.
Chapter 8, Writing a Rendering Engine, will move you into the actual rendering territory and use the thin abstraction layer, which is discussed in Chapter 6, OpenGL ES 3.1 and Cross-platform Rendering, to implement a 3D rendering framework capable of rendering geometry loaded from files using materials, lights, and shadows.
Chapter 9, Implementing Game Logic, introduces a common approach to organize interactions between gaming code and the user interface part of the application. The chapter begins with an implementation of the Boids algorithm and then proceeds with the extension of our user interface implemented in the previous chapters.
Chapter 10, Writing Asteroids Game, continues to put together the material from previous chapters. We will implement an Asteroids game with 3D graphics, shadows, particles, and sounds using techniques and code fragments introduced in the previous chapters.