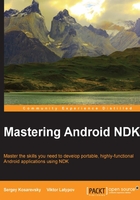
Curl
The libcurl library http://curl.haxx.se/libcurl is a free and easy to use client-side URL transfer library. It is a de facto standard for native applications, which deal with numerous networking protocols. Linux and OS X users enjoy having the library on their system, and a possibility to link against it using the -lcurl
switch. Compilation of libcurl for Android on a Windows host requires some additional steps to be done. We explain them here.
The libcurl library build process is based on autoconf
; we will need to generate the curl_config.h
file before actually building the library. Run the configure script from the folder containing the unpacked libcurl distribution package. Cross-compilation command-line flags should be set to:
--host=arm-linux CC=arm-eabi-gcc
The -I
parameter of the CPPFLAGS
variable should point to the /system/core/include
subfolder of your NDK folder, in our case:
CPPFLAGS="-I D:/NDK/system/core/include"
The libcurl library can be customized in many ways. We use this set of parameters (disable all protocols except HTTP and HTTPS):
>configure CC=arm-eabi-gcc --host=arm-linux --disable-tftp --disable-sspi --disable-ipv6 --disable-ldaps --disable-ldap --disable-telnet --disable-pop3 --disable-ftp --without-ssl --disable-imap --disable-smtp --disable-pop3 --disable-rtsp --disable-ares --without-ca-bundle --disable-warnings --disable-manual --without-nss --enable-shared --without-zlib --without-random --enable-threaded-resolver --with-ssl
The --with-ssl
parameter enables the usage of OpenSSL library to provide secure HTTPS transfers. This library will be discussed further in this chapter. However, in order to work with SSL-encrypted connections, we need to tell libcurl where our system certificates are located. This can be done with CURL_CA_BUNDLE
defined in the beginning of the curl_config.h
file:
#define CURL_CA_BUNDLE "/etc/ssl/certs/ca-certificates.crt"
The configure script will generate a valid curl_config.h
header file. You may find it in the book's source code bundle. Compilation of an Android static library requires a usual set of Android.mk
and Application.mk
files, which is also within the 1_Curl
example. In the next chapter, we will learn how to use the libcurl library to download the actual content from Internet over HTTPS. However, here is a simplistic usage example to retrieve a HTTP page:
CURL* Curl = curl_easy_init(); curl_easy_setopt( Curl, CURLOPT_URL, "http://www.google.com" ); curl_easy_setopt( Curl, CURLOPT_FOLLOWLOCATION, 1 ); curl_easy_setopt( Curl, CURLOPT_FAILONERROR, true ); curl_easy_setopt( Curl, CURLOPT_WRITEFUNCTION, &MemoryCallback ); curl_easy_setopt( Curl, CURLOPT_WRITEDATA, 0 ); curl_easy_perform( Curl ); curl_easy_cleanup( Curl );
Here MemoryCallback()
is a function that handles the received data. It can be as tiny as the following code fragment:
size_t MemoryCallback( void* P, size_t Size, size_t Num, void* ) { if ( !P ) return 0; printf( "%s\n", P ); }
The retrieved data will be printed on the screen in your desktop application. The same code will work like a dummy in Android, without producing any visible side effects, since the printf()
function is just a dummy there.