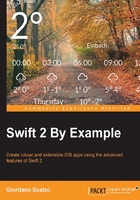
Connecting the dataSource and the delegate
You have probably noticed that when we created collectionview
, we set View Controller itself as dataSource
and delegate
:
collectionView.dataSource = self collectionView.delegate = self
A common pattern found in Cocoa in many components is the delegate pattern, where part of the behavior is delegated to another object, and that object must implement a particular protocol.
In the case of UICollectionView
, and likewise for UITableView
, we have to delegate one of the class references to provide the content for the view, dataSource
, and the other to react to events from the view itself. In this way, the presentation level is completely decoupled from the data and the business logic, which reside in two specialized objects.
So, we need to implement the required methods of the protocols:
// MARK: UICollectionViewDataSource extension MemoryViewController: UICollectionViewDataSource { func collectionView(collectionView: UICollectionView, numberOfItemsInSection section: Int) -> Int { return deck.count } func collectionView(collectionView: UICollectionView, cellForItemAtIndexPath indexPath: NSIndexPath) -> UICollectionViewCell { let cell = collectionView.dequeueReusableCellWithReuseIdentifier("cardCell", forIndexPath: indexPath) cell.backgroundColor = .sunflower() return cell } }
As you can see, in the method called for the cell at a certain position, we are calling a method to reuse a cell instead of creating a new one. This is a nifty feature of UICollectionView
that saves all the cells in a cache and can reuse those outside the visible view. This not only saves a lot of memory, but it is also really efficient because creating new cells during scrolling could be CPU consumption and affect performance.
Because we want to see just the flow of the card, we use the default empty cell as the view cell, changing the color of the background:
// MARK: UICollectionViewDelegate extension MemoryViewController: UICollectionViewDelegate { func collectionView(collectionView: UICollectionView, didSelectItemAtIndexPath indexPath: NSIndexPath) { } }
For the delegate, we simply prepare ourselves to handle a touch on the card. Because we don't need a real deck of cards, an array of integers is enough as a model:
override func viewDidLoad() { super.viewDidLoad() setup() start() } private func start() { deck = Array<Int>(count: numCardsNeededDifficulty(difficulty), repeatedValue: 1) collectionView.reloadData() }
Now, upon running the app and choosing a level, we will have all our empty cards laid out like this:

Using a different simulator and the iPhone 5 or 4S, we can see that our table adapts its size smoothly.
Note
The code for the app implemented so far can be downloaded from https://github.com/gscalzo/Swift2ByExample/tree/2_Memory_2_Cards_Layout.