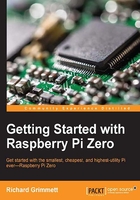
Basic programming constructs on Raspberry Pi Zero
Now that you know how to enter and run a simple Python program on Raspberry Pi Zero, let's look at some more complex programming tools. Specifically, we'll cover what to do when we want to determine the instructions to execute and how to loop our code to do that more than once. I'll give a brief introduction on how to use libraries in the Python version 2.7 code and how to organize statements into functions. Finally, we'll very briefly cover object-oriented code organization.
Note
Indentation in Python is very important; it will specify which group of statements is associated with a given loop or decision set, so watch your indentation carefully.
The if statement
As you have seen in previous examples, your programs normally start by executing the first line of code and then continue with the following lines until the program runs out of code. But, what if you want to decide between two different courses of action? We can do this in Python using an if
statement. The following screenshot shows some example code:

The following are the details of the code shown in the previous screenshot:
#!/usr/bin/python
: This is included so that you can make your program executable.a = input("Input value: ")
: The data will be input by the user and stored ina
.b = input("Input second value: ")
: This data will also be input by the user and stored inb
.if a > b:
: This is anif
statement. The expression evaluated in this case isa > b
. If it isTrue
, the program will execute the next one or more statements that are indented; in this case,c = a - b
. If not, it will skip that statement.else:
:Theelse
statement is an optional part of the command. If the expression in theif
statement is evaluated asFalse
, the indented statements after theelse:
statement will be executed; in this case,c = b - a
.print c
: Another basic purpose of our program is to print out results. Theprint
command prints out the value ofc
.
You can run the previous program a couple of times, checking both the True
and False
possibilities of the if
expression, as shown in the following screenshot:

The while statement
Another useful construct is the while
construct; it allows us to execute a set of statements over and over again until a specific condition has been met. The following screenshot shows a set of code that uses this construct:

The following are the details of the code shown in the previous screenshot:
#!/usr/bin/python
: This is included so you can make your program executable.a = 0
: This line sets the value of variablea
to0
. We'll need this only to make sure that we execute the loop at least once.b = 1
: This line sets the value of the variableb
to1
. We'll need this only to make sure that we execute the loop at least once.while a != b:
: The expressiona != b
(in this case,!=
means not equal to) is verified. If it isTrue
, the indented statements are executed. When the statement is evaluated asFalse
, the program jumps to the statements (none in this example) after the indented section.a = input("Input value: ")
: The data will be input by the user and stored ina
.b = input("Input second value: ")
: This data will also be input by the user and stored inb
.c = a + b
: The variablec
is loaded with the sum ofa
andb
.print c
: Theprint
command prints out the value ofc
.
Now you can run the program. Note that when you enter the same value for a
and b
, the program stops, as shown in the following screenshot:

Working with functions
The next concept that we need to cover is how to put a set of statements into a function. We use functions to organize code and group sets of statements together when it makes sense to organize them in the same location. For example, if we have a specific calculation that we might want to perform many times, instead of copying the set of statements every time we want to perform it, we group them into a function. I'll use a fairly simple example here, but if the calculation takes a significant number of programming statements, you can see how that would make our code significantly easier to maintain, as we don't need to duplicate our code over and over. It can also make our code easier to read. The following screenshot shows the code:

The following is the explanation of the code from our previous example:
#!/usr/bin/python
: This is included so you can make your program executable.def sum(a, b):
: This line defines a function namedsum
. Thesum
function takesa
andb
as arguments.c = a + b
: Whenever this function is called, it will add the values in the variablea
to the values in variableb
.return c
: When the function is executed, it will return variablec
to the calling expression.d = input("Input value: ")
: This data will also be input by the user and will be stored ind
. The promptInput second value:
will be shown to the user.e = input("Input second value: ")
:This data will also be input by the user and stored ine
. The promptInput second value:
will be shown to the user.f = sum(d, e)
: The functionsum
is called. The program then goes to thesum
function and executes it. The value in variabled
is copied to the variablea
and the value in the variablee
is copied to the variableb
. The value is returned from the function and then stored in variablef
.print f
: Theprint
command prints out the value off
.
The following screenshot is the result received when you run the code:

Libraries/modules in Python
The next topic we need to cover is how to add functionality to our programs using libraries/modules. Libraries or modules, as they are sometimes called in Python, include a functionality that someone else has created and that you want to add to your code. As long as the functionality exists and your system knows about it, you can include the library in the code.
So, let's modify our code again by adding the library, as shown in the following screenshot:

The following is a line-by-line description of the code:
#!/usr/bin/python
: This is included so that you can make your program executable.import time
: This includes thetime
library. Thetime
library includes a function that allows you to pause for a specified number of seconds.d = input("Input value: ")
: This data will be input by the user and will be stored ind
. The promptInput second value:
will be shown to the user.time.sleep(2)
: This line calls thesleep
function in thetime
library, which will cause a 2 second delay.e = input("Input second value: ")
: This data will also be input by the user and will be stored inb
. The promptInput second value:
will be shown to the user.f = d + e
: Thef
variable is loaded with the valued + e
.print f
: Theprint
command prints out the value off
.
The following screenshot shows the result after running the previous example code:

Of course, this looks very similar to other results. But you will notice a pause between you entering the first value and the appearance of the second value.