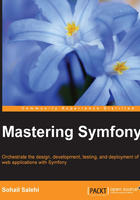
Creating data fixtures
Technically, a data fixture is a PHP class with a few initialized objects. In AppBundle
, create this directory and file structure:
/DataFixtures/ORM/LoadUsers.php
Add the following content to our class:
<?php // mava/src/AppBundle/DataFixtures/ORM/LoadUsers.php namespace AppBundle\DataFixtures\ORM; use Doctrine\Common\DataFixtures\FixtureInterface; use Doctrine\Common\Persistence\ObjectManager; use AppBundle\Entity\User; class LoadUsers implements FixtureInterface { public function load(ObjectManager $manager) { // todo: create and persist objects } }
This is the general structure of a data fixture. As you can see, it implements FixtureInterface
and has a load()
method for data persistence.
All we need to do is create a few objects, set their values, and ask our object manager to persist them:
public function load(ObjectManager $manager) { $user1 = new User(); $user1->setName('John'); $user1->setBio('He is a cool guy'); $user1->setEmail('john@mava.info'); $manager->persist($user1); $user2 = new User(); $user2->setName('Jack'); $user2->setBio('He is a cool guy too'); $user2->setEmail('jack@mava.info'); $manager->persist($user2); $manager->flush(); }
Remember those setters and getters in our User
entity? That's how we use them here. These two objects are all set and ready to be persisted in our database. The flush()
method executes both queries in one shot. This means that we can have multiple queries created and run them in one step. This is the beauty of Doctrine. Now we are all set and ready to load what we have created.
Loading data fixtures
Loading can be done via a simple command:
$ bin/console doctrine:fixtures:load
It will ask you if you want to erase the previous content of the table first. Answer Y and press Enter:
Careful, database will be purged. Do you want to continue Y/N ?Y > purging database > loading mava\CoreBundle\DataFixtures\ORM\LoadUsers
Now check your User
table. As you can see, there are two new records here:
