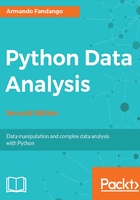
Indexing NumPy arrays with Booleans
Boolean indexing is indexing based on a Boolean array and falls in the family of fancy indexing. Since Boolean indexing is a kind of fancy indexing, the way it works is essentially the same.
The following is the code for this segment (refer to boolean_indexing.py
in this book's code bundle):
import scipy.misc import matplotlib.pyplot as plt import numpy as np face = scipy.misc.face() xmax = face.shape[0] ymax = face.shape[1] face=face[:min(xmax,ymax),:min(xmax,ymax)] def get_indices(size): arr = np.arange(size) return arr % 4 == 0 face1 = face.copy() xindices = get_indices(face.shape[0]) yindices = get_indices(face.shape[1]) face1[xindices, yindices] = 0 plt.subplot(211) plt.imshow(face1) face2 = face.copy() face2[(face > face.max()/4) & (face < 3 * face.max()/4)] = 0 plt.subplot(212) plt.imshow(face2) plt.show()
The preceding code implies that indexing occurs with the aid of a special iterator object.
The following steps will give you a brief explanation of the preceding code:
- Image with dots on the diagonal.
This is in some manner similar to the Fancy indexing section. This time we choose modulo 4 points on the diagonal of the picture:
def get_indices(size): arr = np.arange(size) return arr % 4 == 0
Then, we just use this selection and plot the points:
face1 = face.copy() xindices = get_indices(face.shape[0]) yindices = get_indices(face.shape[1]) face1[xindices, yindices] = 0 plt.subplot(211) plt.imshow(face1)
- Set to
0
based on value.Select array values between one quarter and three quarters of the maximum value and set them to
0
:face2[(face > face.max()/4) & (face < 3 * face.max()/4)] = 0
The diagram with the two new pictures is presented as follows: