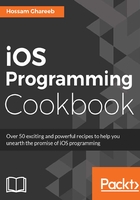
上QQ阅读APP看书,第一时间看更新
How to do it...
- Create a new Xcode project with a Single View template named NavigationController.
- From Object library, drag a navigation controller to the storyboard, and set the entry point of the storyboard to the navigation controller.
- By default, the navigation controller in interface builder comes with a root view controller, which is a UITableViewController. We will use this table to display a list of dates, and when you click on one of them, it will push another view controller.
- Create a new view controller called MasterViewController to be the root view controller and make it extend from UITableViewController. The source code of MasterViewController should look like this:
class MasterViewController: UITableViewController {
var objects = [AnyObject]()
override func viewDidLoad() {
super.viewDidLoad()
let addButton = UIBarButtonItem(barButtonSystemItem: .Add,
target: self, action: "insertNewObject:")
self.navigationItem.rightBarButtonItem = addButton
}
func insertNewObject(sender: AnyObject) {
objects.insert(NSDate(), atIndex: 0)
let indexPath = NSIndexPath(forRow: 0, inSection: 0)
self.tableView.insertRowsAtIndexPaths([indexPath],
withRowAnimation: .Automatic)
}
// MARK: - Segues
override func prepareForSegue(segue: UIStoryboardSegue, sender:
AnyObject?) {
if segue.identifier == "showDetail" {
if let indexPath = self.tableView.indexPathForSelectedRow {
let object = objects[indexPath.row] as! NSDate
let controller = segue.destinationViewController as!
DetailViewController
controller.detailItem = object
}
}
}
// MARK: - Table View
override func tableView(tableView: UITableView, numberOfRowsInSection
section: Int) -> Int {
return objects.count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath
indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell",
forIndexPath: indexPath)
let object = objects[indexPath.row] as! NSDate
cell.textLabel!.text = object.description
return cell
}
}
- Then, set the class of the root view controller in the storyboard to be MasterViewController from Identity Inspector.
- Add a new view controller in Xcode called DetailViewController to display the details when you select an item from MasterViewController:
class DetailViewController: UIViewController {
@IBOutlet weak var detailDescriptionLabel: UILabel!
var detailItem: AnyObject? {
didSet {
// Update the view.
self.configureView()
}
}
func configureView() {
// Update the user interface for the detail item.
if let detail = self.detailItem {
if let label = self.detailDescriptionLabel {
label.text = detail.description
}
}
}
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from
a nib.
self.configureView()
}
}
- Add a new view controller in the storyboard, and set its class to DetailViewController.
- From the prototype cell of the table view in MasterViewController, hold the Ctrl key, create a segue to the DetailViewController, and select show as a type of the segue.
- Click on the segue, and set the identifier in the Attribute Inspector to showDetail:
- Add a UILabel as a subview to display the details of the selected item and connect the outlet to source code of DetailViewController.swift:
- Lastly, in the MasterViewController in the storyboard, you will see a table view that has a prototype cell. Update the identifier of the cell to Cell to match the identifier in the source code.
If you are not familiar with table views, don't worry, we will explain them in detail in later chapters.
- Now, build and run. You will see an empty list in MasterViewController; click on the add button multiple times; you will see something like this:
- Also, when you click on any item, it should navigate you to DetailViewController: