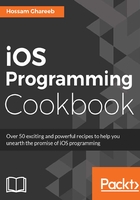
上QQ阅读APP看书,第一时间看更新
How to do it...
Here, we will create the stack data structure with/without generics:
- Create a new playground named Generics.
- Let's create the data structure stack with type Int:
class StackInt{ var elements = [Int]() func push(element:Int) { self.elements.append(element) } func pop() ->Int { return self.elements.removeLast() } func isEmpty()->Bool { returnself.elements.isEmpty } } var stack1 = StackInt() stack1.push(5) // [5] stack1.push(10) //[5,10] stack1.push(20) // [5,10,20] stack1.pop() // 20
- Let's see the same created stack but with a generics fashion:
class Stack <T>{ var elements = [T]() func push(element:T) { self.elements.append(element) } func pop()->T{ return self.elements.removeLast() } } var stackOfStrings = Stack<String>() stackOfStrings.push("str1") stackOfStrings.push("str2") stackOfStrings.pop() var stackOfInt = Stack<Int>() stackOfInt.push(4) stackOfInt.push(7) stackOfInt.pop()