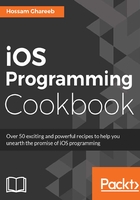
Enums with associated values
Last but not least, we will talk about another feature in Swift enums, which is creating enums with associated values. Associated values let you store extra information with enum case value. Let's take a look at the problem and how we can solve it using associated values in enums.
Suppose we are working with an app that works with products, and each product has a code. Some products codes are represented by QR code format, but others by UPC format. Check out the following image to see the differences between two codes at http://www.mokemonster.com/websites/erica/wp-content/uploads/2014/05/upc_qr.png):
The UPC code can be represented by four integers; however, QR code can be represented by a string value. To create an enum to represent these two cases, we would do something like this:
enum ProductCode{ case UPC(Int, Int, Int, Int) case QR(String) } var productCode = ProductCode.UPC(4, 88581, 1497, 3) productCode = ProductCode.QR("BlaBlaBla")
First, UPC is a case, which has four integer values, and the second is a QR, which has a string value. Then, you can create enums the same way we created before in other enums, but here you just have to pass parameters for the enum. When you need to check the value of enum with its associated value, we will use a switch statement as usual, but with some tweaks:
switch productCode{ case .UPC(let numberSystem, let manufacturerCode, let productCode, let checkDigit): print("Product UPC code is \(numberSystem) \(manufacturerCode) \(productCode) \(checkDigit)") case .QR(let QRCode): print("Product QR code is \(QRCode)") } // "Product QR code is BlaBlaBla"