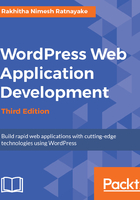
The best action for adding user roles
The user roles discussed in the previous section will be saved in the database as settings. Therefore, only a single call to this function is required throughout the life cycle of an application. We have two options for implementing this functionality. Application installation and plugin activation are the most suitable places to call these kinds of functions to eliminate duplicate executions:
- Application installation: WordPress provides a well-defined step-by-step installation process. Similarly, every application or plugin needs an installation process. Once installation is completed, these files are not accessible again. Therefore, plugin installation is the ideal place to create user roles.
- Plugin activation: WordPress plugin activation hooks let us execute certain functionality on plugin activation. This is also a one-time process in a plugin. However, we can deactivate and reactivate the plugin multiple times. So, this functionality gets executed multiple times and hence we have to check whether it's already been executed. If this is not checked, all the changes made after the activation will be reset to default values. So, plugin activation is the second-best option for this type of functionality.
Here, we will be using plugin activation to add and remove application user roles since we don't have an application installation feature at this stage.
First, we need to identify where we can add the activation-related functionality. Basically, these are configurations of the forum application. Therefore, we need to create a new class called WPWAF_Config_Manager inside a new folder called classes, in our main plugin. Next, we have to initialize an object from this class inside the instance function of the WPWAF_Forum class using the following code:
self::$instance->config_manager = new WPWAF_Config_Manager();
Then we need to include the class file inside the includes function of the WPWAF_Forum class using the following code:
require_once WPWAF_PLUGIN_DIR . 'classes/class-wpwaf-config-manager.php';
Now we can include the activation hook inside the instance function, as shown in the following code:
register_activation_hook( __FILE__, array( self::$instance- >config_manager , 'activation_handler' ) );
The activation_handler function of the WPWAF_Config_Manager class will be responsible for handling all functionality related to forum plugin activation. Let's look at the add_application_user_roles function inside the config class, as shown in the following code:
class WPWAF_Config_Manager{
public function activation_handler(){
$this->add_application_user_roles();
}
public function add_application_user_roles(){
add_role( 'wpwaf_premium_member', __('Premium Member','wpwaf'), array( 'read' => true ) );
add_role( 'wpwaf_free_member', __('Free Member','wpwaf'), array( 'read' => true ) );
add_role( 'wpwaf_moderator', __('Moderator','wpwaf'), array( 'read' => true ) );
}
}
The register_activation_hook function will be called when the plugin is activated and hence avoids duplicate calls to the database.
You might have noticed that all three user roles of the application are created with the read capability. WordPress is built to create websites and hence most of the default capabilities will be related to CMS features. In web applications, we need custom capabilities more often than not. Therefore, we can keep the basic read capability and add new custom capabilities as we move on. All the users will get a very basic admin area containing a dashboard and profile information, as shown in the following screenshot:
