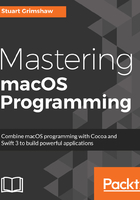
上QQ阅读APP看书,第一时间看更新
Data flow
We will maintain as little state as possible. We will have properties only for values that must persist beyond function calls, so we will try to replace something like this:
someProperty = propertyValue
functionThatUsesSomeProperty()
With this, wherever possible:
functionThatUses(someProperty: propertyValue)
In the same vein, we will prefer to replace functions that access a property like this:
let customerName = "Sally"
let customerID = 101
func customerDetails() -> String
{
return customerName
+ "\n\(customerID)"
}
let customerDetailsString = customerDetails()
With functions that take arguments explicitly, like this:
func betterCustomerDetails(
customerName: String,
customerID: Int) -> String
{
return customerName
+ "\n\(customerID)"
}
let betterCustomerDetailsString = betterCustomerDetails(
customerName: "Sally",
customerID: 101)
This way, we gain clarity in two places. Firstly, the function's signature shows explicitly that something is to be done with a customerName and customerID value. Secondly, we can see at the call site what data is required by the function.
Yes, there's a little more typing to do. This is a tiny price to pay for increased clarity, especially five months from now, when your app is being bought for a zillion dollars by Facebook, and they want you to refactor it.
This is the sort of stuff you'll pick up as you develop functional programming habits. Or read this book. Or best of all, both.