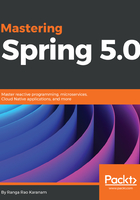
上QQ阅读APP看书,第一时间看更新
Defining a HandlerInterceptor
The following are the methods you can override in HandlerInterceptorAdapter:
- public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler): Invoked before the handler method is invoked
- public void postHandle(HttpServletRequest request, HttpServletResponse response, Object handler, ModelAndView modelAndView): Invoked after the handler method is invoked
- public void afterCompletion(HttpServletRequest request, HttpServletResponse response, Object handler, Exception ex): Invoked after the request processing is complete
The following example implementation shows how to create a HandlerInterceptor. Let's start with creating a new class that extends HandlerInterceptorAdapter:
public class HandlerTimeLoggingInterceptor extends
HandlerInterceptorAdapter {
The preHandle method is invoked before the handler is called. Let's place an attribute on the request, indicating the start time of handler invocation:
@Override
public boolean preHandle(HttpServletRequest request,
HttpServletResponse response, Object handler) throws Exception {
request.setAttribute(
"startTime", System.currentTimeMillis());
return true;
}
The postHandle method is invoked after the handler is called. Let's place an attribute on the request, indicating the end time of the handler invocation:
@Override
public void postHandle(HttpServletRequest request,
HttpServletResponse response, Object handler,
ModelAndView modelAndView) throws Exception {
request.setAttribute(
"endTime", System.currentTimeMillis());
}
The afterCompletion method is invoked after the request processing is complete. We will identify the time spent in the handler using the attributes that we set into the request earlier:
@Override
public void afterCompletion(HttpServletRequest request,
HttpServletResponse response, Object handler, Exception ex)
throws Exception {
long startTime = (Long) request.getAttribute("startTime");
long endTime = (Long) request.getAttribute("endTime");
logger.info("Time Spent in Handler in ms : "
+ (endTime - startTime));
}