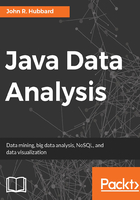
Hash tables
A dataset of key-value pairs is usually implemented as a hash table. It is a data structure in which the key acts like an index into the set, much like page numbers in a book or line numbers in a table. This direct access is much faster than sequential access, which is like searching through a book page-by-page for a certain word or phrase.
In Java, we usually use the java.util.HashMap<Key,Value>
class to implement a key-value pair dataset. The type parameters Key
and Value
are specified classes. (There is also an older HashTable
class, but it is considered obsolete.)
Here is a data file of seven South American countries:

Figure 2-1 Countries data file
Here is a Java program that loads this data into a HashMap
object:

Listing 2-1 HashMap example for Countries data
The Countries.dat
file is in the data
folder. Line 15 instantiates a java.io.File
object named dataFile
to represent the file. Line 16 instantiates a java.util.HashMap
object named dataset
. It is structured to have String
type for its key and Integer
type for its value. Inside the try
block, we instantiate a Scanner
object to read the file. Each data point is loaded at line 22, using the put()
method of the HashMap
class.
After the dataset has been loaded, we print its size at line 27 and then print the value for Peru
at line 28. (The format code %,d
prints the integer value with commas.)
Here is the program's output:

Figure 2-2 Output from HashMap program
Notice, in the preceding example, how the get()
method implements the input-output nature of the hash table's key-value structure. At line 28, the input is the name Peru
and the resulting output is its population 29,907,003
.
It is easy to change the value of a specified key in a hash table. Here is the same HashMap
example with these three more lines of code added:

Listing 2-2 HashMap example revised
Line 29 changes the value for Peru
to be 31,000,000
.
Here's the output from this revised program:

Figure 2-3 Output from revised HashMap program
Notice that the size of the hash table remains the same. The put()
method adds a new data point only when the key value is new.