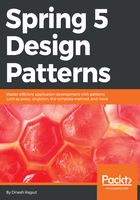
Implementing the Facade design pattern
Let's look into the following listings to demonstrate the Facade design pattern.
Create subsystem service classes for your Bank application: Let's see the following PaymentService class for the subsystem.
Following is the PaymentService.java file:
package com.packt.patterninspring.chapter3.facade.pattern; public class PaymentService { public static boolean doPayment(){ return true; } }
Let's create another service class AccountService for the subsystem.
Following is the AccountService.java file:
package com.packt.patterninspring.chapter3.facade.pattern; import com.packt.patterninspring.chapter3.model.Account; import com.packt.patterninspring.chapter3.model.SavingAccount; public class AccountService { public static Account getAccount(String accountId) { return new SavingAccount(); } }
Let's create another service class TransferService for the subsystem.
Following is the TransferService.java file:
package com.packt.patterninspring.chapter3.facade.pattern; import com.packt.patterninspring.chapter3.model.Account; public class TransferService { public static void transfer(int amount, Account fromAccount,
Account toAccount) { System.out.println("Transfering Money"); } }
Create a Facade Service class to interact with the subsystem: Let's see the following Facade interface for the subsystem and then implement this Facade interface as a global banking service in the application.
Following is the BankingServiceFacade.java file:
package com.packt.patterninspring.chapter3.facade.pattern; public interface BankingServiceFacade { void moneyTransfer(); }
Following is the BankingServiceFacadeImpl.java file:
package com.packt.patterninspring.chapter3.facade.pattern; import com.packt.patterninspring.chapter3.model.Account; public class BankingServiceFacadeImpl implements
BankingServiceFacade{ @Override public void moneyTransfer() { if(PaymentService.doPayment()){ Account fromAccount = AccountService.getAccount("1"); Account toAccount = AccountService.getAccount("2"); TransferService.transfer(1000, fromAccount, toAccount); } } }
Create the client of the Facade:
Following is the FacadePatternClient.java file:
package com.packt.patterninspring.chapter3.facade.pattern; public class FacadePatternClient { public static void main(String[] args) { BankingServiceFacade serviceFacade = new
BankingServiceFacadeImpl(); serviceFacade.moneyTransfer(); } }